python读取txt文件,根据条件筛选数据并且生成图像
时间: 2024-11-18 08:16:51 浏览: 16
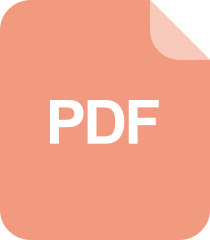
python读取文件名称生成list的方法
在Python中,我们可以使用内置的`open()`函数以及`csv`模块或者第三方库如`pandas`来读取.txt文本文件,然后通过条件筛选数据。如果你的数据是以逗号分隔值(CSV)形式存储的,可以使用`pandas`更方便。以下是基本步骤:
1. **导入必要的库**:
```python
import pandas as pd
import matplotlib.pyplot as plt
```
2. **读取txt文件**,如果文件是纯文本格式,使用`open()`:
```python
with open('file.txt', 'r') as file:
lines = file.readlines()
data_list = [line.strip() for line in lines]
```
3. **如果数据是CSV,使用pandas**:
```python
df = pd.read_csv('file.txt', delimiter='\t' or ',' if comma_separated else None) # 判断分隔符
```
4. **筛选数据**:
```python
filtered_data = df[df[column_name] >= condition] # column_name是你想要筛选的列名,condition是筛选条件
```
5. **处理并绘制图像**:
- 对于数值数据,可以用matplotlib画折线图、柱状图等:
```python
filtered_data.plot(kind='bar') # 或者用其他kind参数
plt.show()
```
6. **针对文本数据**,如果想基于特定关键词生成词云图,可以使用`wordcloud`库:
```python
from wordcloud import WordCloud
text = ' '.join(filtered_data['column_name'])
wordcloud = WordCloud().generate(text)
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis("off")
plt.show()
```
注意替换上述代码中的`file.txt`、`column_name`、`condition`和`delimiter`为实际文件路径、列名、筛选条件和合适的分隔符。
阅读全文
相关推荐
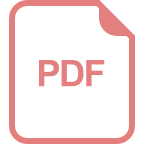
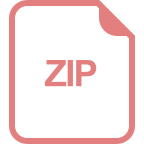















