帮我用C++编写一个俄罗斯方块小游戏
时间: 2023-08-21 10:01:38 浏览: 126
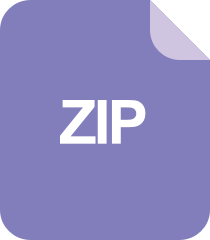
用最简单的C++实现的简易俄罗斯方块

好的,以下是一个简单的俄罗斯方块小游戏的代码实现,供参考:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <conio.h>
#include <windows.h>
using namespace std;
const int WIDTH = 12;
const int HEIGHT = 20;
char gameBoard[HEIGHT][WIDTH] = {0};
int currentX = 5, currentY = 0;
int currentShape = 0, currentRotation = 0;
int score = 0;
// 俄罗斯方块的七种形状
const char shapes[7][4][5] = {
{
"....",
".xx.",
".xx.",
"...."
},
{
"....",
"..x.",
".xxx",
"...."
},
{
"....",
".xx.",
"..xx",
"...."
},
{
"....",
"..x.",
"..x.",
".xx."
},
{
"....",
"..x.",
".xx.",
".x.."
},
{
"....",
".x..",
".xx.",
"..x."
},
{
"....",
".x..",
".xxx",
"...."
}
};
void initGameBoard()
{
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (i == HEIGHT - 1 || j == 0 || j == WIDTH - 1) {
gameBoard[i][j] = '#';
} else {
gameBoard[i][j] = ' ';
}
}
}
}
void printGameBoard()
{
system("cls");
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
cout << gameBoard[i][j];
}
cout << endl;
}
cout << "Score: " << score << endl;
}
void generateNewShape()
{
srand((unsigned)time(NULL));
currentShape = rand() % 7;
currentRotation = rand() % 4;
currentX = 5;
currentY = 0;
}
bool canMove(int x, int y, int rotation)
{
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (shapes[currentShape][i][j] != '.') {
int newX = x + j;
int newY = y + i;
if (newX < 1 || newX >= WIDTH - 1 || newY < 0 || newY >= HEIGHT || gameBoard[newY][newX] != ' ') {
return false;
}
}
}
}
return true;
}
void moveShape(int deltaX, int deltaY, int deltaRotation)
{
if (canMove(currentX + deltaX, currentY + deltaY, (currentRotation + deltaRotation) % 4)) {
currentX += deltaX;
currentY += deltaY;
currentRotation = (currentRotation + deltaRotation) % 4;
}
}
void updateGameBoard()
{
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (shapes[currentShape][i][j] != '.') {
int newX = currentX + j;
int newY = currentY + i;
gameBoard[newY][newX] = shapes[currentShape][i][j];
}
}
}
}
bool isLineFull(int line)
{
for (int i = 1; i < WIDTH - 1; i++) {
if (gameBoard[line][i] == ' ') {
return false;
}
}
return true;
}
void removeLine(int line)
{
for (int i = line; i > 0; i--) {
for (int j = 1; j < WIDTH - 1; j++) {
gameBoard[i][j] = gameBoard[i - 1][j];
}
}
score += 10;
}
void checkFullLines()
{
for (int i = 0; i < HEIGHT - 1; i++) {
if (isLineFull(i)) {
removeLine(i);
}
}
}
int main()
{
initGameBoard();
generateNewShape();
while (true) {
printGameBoard();
updateGameBoard();
Sleep(500);
if (kbhit()) {
char ch = getch();
switch (ch) {
case 'a':
moveShape(-1, 0, 0);
break;
case 'd':
moveShape(1, 0, 0);
break;
case 's':
moveShape(0, 1, 0);
break;
case 'q':
moveShape(0, 0, -1);
break;
case 'e':
moveShape(0, 0, 1);
break;
case 'p':
return 0;
default:
break;
}
} else {
if (canMove(currentX, currentY + 1, currentRotation)) {
currentY++;
} else {
checkFullLines();
generateNewShape();
if (!canMove(currentX, currentY, currentRotation)) {
printGameBoard();
cout << "Game over!" << endl;
return 0;
}
}
}
}
return 0;
}
```
这个实现比较简单,没有考虑很多细节问题,比如说方块落到底部后不能再旋转等。但是这个代码可以让你初步了解俄罗斯方块游戏的实现方式。
阅读全文
相关推荐
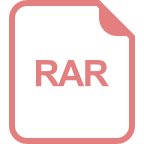

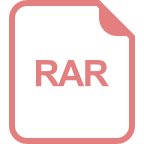
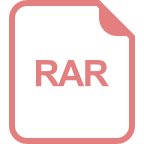
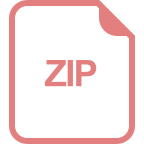
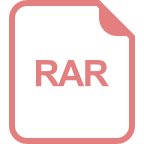
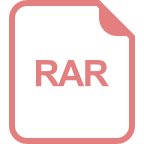
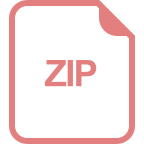
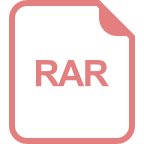
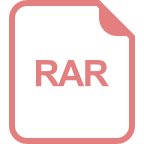
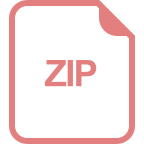
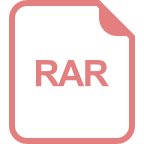
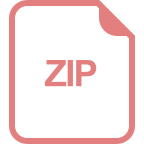
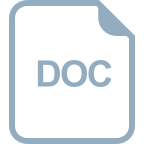
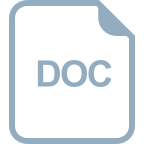

