使用C++11编写合并多个文件夹下的文件的功能的程序
时间: 2024-02-06 13:09:38 浏览: 28
以下是一个使用C++11编写的合并多个文件夹下文件的程序示例,该程序使用了递归遍历文件夹的方法:
```cpp
#include <iostream>
#include <fstream>
#include <filesystem>
#include <string>
namespace fs = std::filesystem;
void mergeFiles(const std::string& sourceDir, const std::string& outputFile) {
std::ofstream out(outputFile);
if (!out) {
std::cerr << "Failed to open output file: " << outputFile << std::endl;
return;
}
for (const auto& entry : fs::recursive_directory_iterator(sourceDir)) {
if (entry.is_regular_file()) {
std::ifstream in(entry.path());
if (!in) {
std::cerr << "Failed to open input file: " << entry.path() << std::endl;
} else {
out << in.rdbuf();
in.close();
}
}
}
out.close();
}
int main() {
std::string sourceDir = "path/to/source/directory";
std::string outputFile = "path/to/output/file.txt";
mergeFiles(sourceDir, outputFile);
return 0;
}
```
要使用此程序,只需要将 `sourceDir` 和 `outputFile` 变量设置为正确的目录和文件路径即可。此程序将递归遍历 `sourceDir` 目录及其子目录,将所有找到的文件合并到 `outputFile` 中。
相关推荐
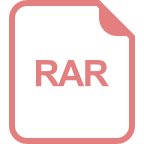
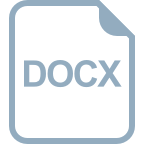
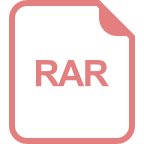














