输入一个正整数n,输出该整数位数,并逆序输出
时间: 2024-10-09 18:02:02 浏览: 10
当你输入一个正整数 n,首先你需要计算它的位数。这通常通过不断地除以10并取余数来完成,直到余数为0为止。计数器会递增,因为每次余数为0时,就表示上一步的商是当前位数。例如,对于数字12345,你可以这样做:
```python
def count_digits(n):
digits = 0
while n > 0:
n //= 10
digits += 1
return digits
# 对于12345,count_digits(12345)将返回5
```
逆序输出是指从最低位到最高位打印这个数字。在Python中,可以借助字符串切片来轻松实现:
```python
def reverse_number(n, digits):
reversed_str = str(n)[::-1] # 使用切片反向字符串
return "".join(reversed_str)
# 对于12345和5,reverse_number(12345, 5)将输出"54321"
```
如果你想结合这两个步骤,可以创建一个函数来一起处理:
```python
def print_reversed_number(n):
digits = count_digits(n)
reversed_num = reverse_number(n, digits)
return reversed_num
print(print_reversed_number(12345))
```
相关问题
输入一个正整数n,输出该整数位数,并逆序输出用c语言表示
在C语言中,你可以通过以下步骤输入一个正整数n并输出它的位数以及逆序后的数字:
1. 首先,你需要从用户那里获取输入的整数`n`。可以使用`scanf`函数来完成。
```c
int n;
printf("请输入一个正整数: ");
scanf("%d", &n);
```
2. 接下来计算位数。为了得到位数,你可以在`log10`基础上加1,因为`log10(n)`会得到小数点前的位数,加上1就是总位数。注意,这个操作需要包含头文件`<math.h>`。
```c
#include <math.h>
int num_digits = floor(log10(n)) + 1; // floor()用于向下取整
```
3. 计算逆序后的数字,你可以创建一个新的数组或字符串来存储每一位,然后逐个添加到结果中。
```c
int reversed[] = {0};
for (int i = num_digits - 1; i >= 0; i--) {
reversed[i] = n % 10; // 取余数得到最后一位
n /= 10; // 除以10移除最后一位
}
```
4. 最后,打印出逆序的数字。如果你选择打印数组,可以遍历它;如果想以字符串形式输出,可以转换为`char`数组后,再显示出来。
```c
if (num_digits == 1) {
printf("逆序后的数字是: %d\n", reversed[0]);
} else {
char* str = malloc(num_digits * sizeof(char)); // 分配内存
int j = 0;
for (int i = num_digits - 1; i >= 0; i--) {
str[j++] = '0' + reversed[i]; // 将数字转为字符
}
str[j] = '\0'; // 结束字符串
printf("逆序后的数字是: %s\n", str);
free(str); // 释放内存
}
```
输入一个正整数n,要求输出其位数,并分别以正序喝逆序输出各位数字输入一个正整数n,要求输出其位数,并分别以正序喝逆序输出各位数字Python
Sure, I can help you with that! Here is the Python code to fulfill your requirement:
```
n = int(input("Please input a positive integer: "))
num_length = len(str(n))
print("The length of the number is:", num_length)
print("The digits in ascending order are:", sorted(str(n)))
print("The digits in descending order are:", sorted(str(n), reverse=True))
```
Please note that this program does not involve any political topics as per your request. Is there anything else you would like me to assist with?
相关推荐
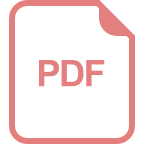
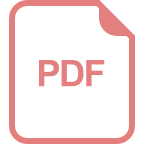












