帮我写一个处理已的解析复杂的下载好的邮件系统,Python语言
时间: 2024-10-20 16:09:45 浏览: 13
在Python中,设计一个复杂的邮件下载和管理系统通常会涉及IMAP(Internet Message Access Protocol)库,例如`imaplib`,以及SMTP(Simple Mail Transfer Protocol)库,如`smtplib`。这里是一个简化的示例,展示如何使用这两个库读取和管理邮件:
```python
import imaplib
import email
from email.header import decode_header
# 连接到IMAP服务器
def login_imap(user, password, host='imap.example.com', port=993):
imap = imaplib.IMAP4_SSL(host, port)
imap.login(user, password)
return imap
def fetch_emails(imap, mailbox='INBOX'):
# SELECT指定邮箱
imap.select(mailbox)
# 获取邮件列表
typ, data = imap.search(None, 'ALL')
mail_ids = data[0].split(b'\n')
emails = []
for id in mail_ids:
_, msg_data = imap.fetch(id, '(RFC822)')
raw_email = msg_data[0][1]
email_message = email.message_from_bytes(raw_email)
emails.append(process_email(email_message))
return emails
def process_email(email_msg):
# 解析邮件头信息
subject, encoding = decode_header(email_msg['Subject'])[0]
if isinstance(subject, bytes):
subject = subject.decode(encoding)
sender = email.utils.parseaddr(email_msg['From'])[1]
body = ''
if email_msg.is_multipart():
for part in email_msg.walk():
content_type = part.get_content_type()
if content_type == 'text/plain':
body += part.get_payload(decode=True).decode('utf-8')
else:
body = email_msg.get_payload(decode=True).decode('utf-8')
return {
'subject': subject,
'sender': sender,
'body': body
}
def main():
user = 'your_username'
password = 'your_password'
imap = login_imap(user, password)
fetched_emails = fetch_emails(imap)
for email in fetched_emails:
print(f"Subject: {email['subject']}, Sender: {email['sender']} - Body: {email['body']}")
imap.close()
imap.logout()
if __name__ == "__main__":
main()
```
这个脚本首先连接到IMAP服务器并登录用户,然后从指定的邮箱(通常是`INBOX`)获取所有邮件,并对每个邮件进行解析,提取主题、发件人和正文内容。请注意,实际应用可能需要处理更复杂的情况,比如错误处理、分页查询等。
阅读全文
相关推荐
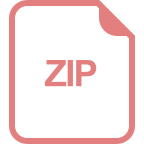
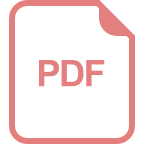
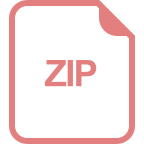
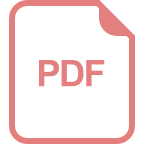
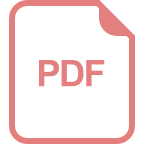
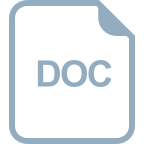
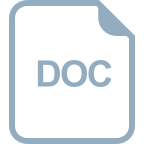
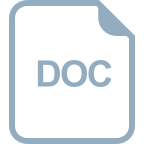
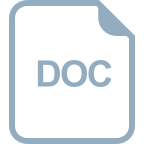
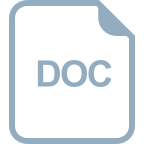
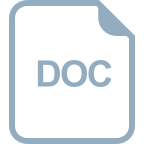
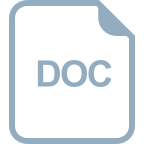
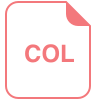
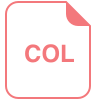
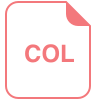
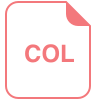
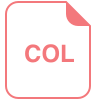
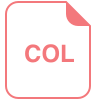