mapper写update
时间: 2024-11-09 19:26:15 浏览: 8
在MyBatis中,Mapper用于处理数据库的CRUD操作,其中Update是修改数据的操作。当你需要更新数据库表中的记录时,你可以编写一条Mapper XML文件或者使用注解的方式,在Java代码中声明更新的方法。
以下是XML映射文件中的基本结构,假设你要更新名为`users`的表中`id`为1的用户信息:
```xml
<update id="updateUser" parameterType="com.example.User">
UPDATE users
SET name = #{name}, email = #{email}
WHERE id = #{id}
</update>
```
在这个例子中,`id`, `name`, 和 `email` 是参数占位符,对应于User对象中的属性。在Java代码里,你会像这样调用这个方法:
```java
User userToUpdate = new User();
userToUpdate.setId(1);
userToUpdate.setName("New Name");
userToUpdate.setEmail("new@email.com");
UserMapper mapper = sqlSession.getMapper(UserMapper.class);
int rowsAffected = mapper.updateUser(userToUpdate);
```
如果你使用的是注解形式,可以在Mapper接口上声明一个方法,例如:
```java
@Mapper
public interface UserMapper {
@Update("UPDATE users SET name = #{name}, email = #{email} WHERE id = #{id}")
int updateUser(@Param("id") int id, @Param("name") String name, @Param("email") String email);
}
```
然后在业务服务类中调用该方法:
```java
User userToUpdate = ...;
int result = userMapper.updateUser(userToUpdate.getId(), userToUpdate.getName(), userToUpdate.getEmail());
```
阅读全文
相关推荐
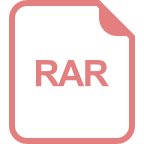
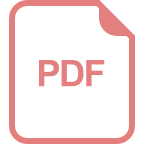
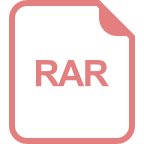
















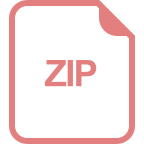