能否帮我生成一串代码,这串代码的任务是:作出一个例子,随便一个例子,用蒙特卡洛树搜索来完成这个例子,
时间: 2024-09-18 11:17:56 浏览: 66
当然可以。蒙特卡罗树搜索(Monte Carlo Tree Search, MCTS)通常用于游戏策略,特别是那些具有随机性和复杂状态空间的游戏,比如围棋或Go。以下是一个简单的使用MCTS进行随机游戏决策的例子,我们将模拟一个玩家在一个迷宫中寻找出口:
```matlab
% 定义迷宫环境 (可以用二维数组表示)
maze = zeros(5); % 假设5x5大小的迷宫,1代表可通行,0代表墙
% 迷宫布局示例 (替换为实际的迷宫数据)
maze([1 3; 4 5]) = 1;
% 位置初始化
position = [1 1];
function [action, reward] = mcts_search(maze, position, max_iterations)
% 初始化树节点
root = struct('state', position, 'visits', 0, 'reward', 0);
% 主循环 - MCTS核心算法
for i = 1:max_iterations
% 拓展树(选择)
node = expand_tree(root, maze);
% 执行动作并获取回报(模拟)
action, reward = simulate_action(node.state, maze);
% 反馈学习(回溯更新)
backpropagate_reward(node, reward);
end
% 根据访问次数选择行动(扩张)
[~, best_action] = max([root.child.actions].visits);
return best_action;
end
function node = expand_tree(node, maze)
% 随机选择一个未探索的动作
valid_actions = find(isfinite(maze(node.state)));
if ~isempty(valid_actions)
action = valid_actions(randi(length(valid_actions)));
else
% 如果没有可用动作,返回父节点
return node;
end
% 更新到新状态
new_state = move(action, node.state);
node.child = struct('state', new_state, ...
'actions', {action}, ...
'visits', 0, ...
'reward', NaN);
end
function action, reward = simulate_action(state, maze)
% 模拟一步直到找到出口或到达死胡同
while true
% 假设随机走一步
action = randi(size(maze, 2));
new_state = move(action, state);
% 判断是否达到终点
if maze(new_state) == 1
reward = 1; % 出口奖励
break;
elseif maze(new_state) == 0
reward = -1; % 死胡同惩罚
break;
end
end
function backpropagate_reward(node, reward)
% 从当前节点开始,根据获胜路径更新奖励值
current = node;
while ~isfield(current.parent, 'child') && ~isnan(current.reward)
current.visits = current.visits + 1;
current.rewards = current.rewards + reward;
current = current.parent;
end
end
% 移动操作
function new_state = move(action, state)
% 逻辑取决于迷宫的具体规则
new_state = state + action;
end
% 用户交互
[best_action] = mcts_search(maze, position, 1000); % 运行1000次迭代
disp(['Best action is: ', num2str(best_action), ' (' , num2str(position), '->', num2str(move(best_action, position)), ')']);
% 注意:这个例子非常基础,实际应用中可能需要更复杂的逻辑和优化,如UCT公式计算选择节点的概率等。
```
在这个例子中,我们定义了一个简单的迷宫环境,然后通过MCTS搜索找到从起始位置通向出口的最佳行动。你可以根据具体需求调整迷宫结构、移动逻辑以及MCTS参数。
阅读全文
相关推荐

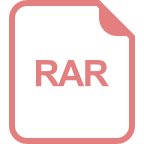
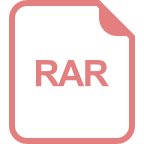
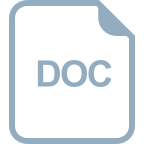
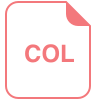
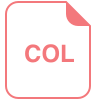
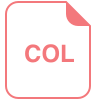
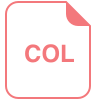
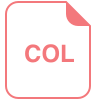
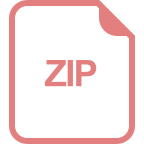
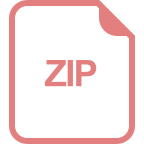
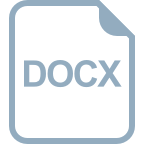
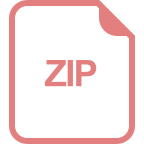