Java贪心算法解题技巧大公开:金融工程与数据压缩技术应用
发布时间: 2024-08-29 17:49:44 阅读量: 53 订阅数: 31 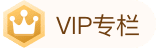
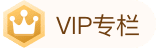
# 1. 贪心算法概述与核心原理
## 1.1 贪心算法的定义与特点
贪心算法是一种在每一步选择中都采取在当前状态下最好或最优(即最有利)的选择,从而希望导致结果是全局最好或最优的算法。其特点在于局部最优解的累加可能会得到全局最优解,但这种策略并不总能成功。
## 1.2 贪心算法的工作原理
贪心算法的工作原理是通过迭代来做出一系列决策,每一决策都选取当前状态下的最优解。其核心在于如何定义“最优”,并据此在问题的所有可能的解中选择出最优的解。
## 1.3 贪心算法的适用条件
贪心算法适用于具有“贪心选择性质”的问题,即局部最优解能被扩展为全局最优解。常见于一些求最大最小值、最短路径等问题中,如哈夫曼编码、图论中的最小生成树和最短路径等。
在设计贪心算法时,需要仔细考虑问题的结构,确保算法的正确性。另外,对于某些问题,贪心算法可能只会得到次优解,因此,在应用贪心算法前需要对其适用性进行深入分析。
# 2. 贪心算法在金融工程中的应用
### 2.1 贪心策略在金融市场分析中的角色
#### 2.1.1 基于贪心算法的交易模型构建
在金融市场分析中,贪心算法可以用来构建交易模型,通过不断地选择局部最优解,以期望获得全局最优的交易策略。构建此类模型的步骤如下:
1. **市场数据收集**:收集历史价格、交易量、财务报告等信息。
2. **特征工程**:选择或计算关键特征,如股价变动率、交易量变化、市盈率等。
3. **策略生成**:定义贪心规则,如买卖信号的生成条件。
4. **回测模拟**:利用历史数据测试策略的有效性。
5. **参数调优**:通过模拟结果调整策略参数,以达到更好的性能。
例如,一个简单的基于移动平均线的交易模型可以使用贪心算法来构建,其中当短期移动平均线向上穿过长期移动平均线时,发出买入信号;反之,发出卖出信号。
**代码示例**:
```java
// Java代码:简单的移动平均交易模型
public class MovingAverageStrategy {
private List<Double> prices;
private int shortTermMA;
private int longTermMA;
public MovingAverageStrategy(List<Double> prices, int shortTermMA, int longTermMA) {
this.prices = prices;
this.shortTermMA = shortTermMA;
this.longTermMA = longTermMA;
}
public boolean shouldBuy() {
double shortMA = calculateMA(prices, shortTermMA);
double longMA = calculateMA(prices, longTermMA);
return shortMA > longMA;
}
public boolean shouldSell() {
double shortMA = calculateMA(prices, shortTermMA);
double longMA = calculateMA(prices, longTermMA);
return shortMA < longMA;
}
private double calculateMA(List<Double> prices, int period) {
double sum = 0;
for (int i = prices.size() - 1; i >= period; i--) {
sum += prices.get(i);
}
return sum / period;
}
}
```
在这个例子中,`shouldBuy` 和 `shouldSell` 方法利用移动平均线来决定买卖时机,这就是一种贪心策略。
#### 2.1.2 风险评估与管理
使用贪心算法进行风险评估与管理通常涉及到确定最优的风险-收益比。在金融工程中,贪心策略可以帮助我们识别可能的投资机会和风险点。这通常涉及到以下步骤:
1. **风险指标计算**:使用如VaR(风险价值)、ES(预期短缺)等指标。
2. **投资组合优化**:选择最优投资组合以达到最大收益同时最小化风险。
3. **动态调整**:根据市场变化,调整投资组合以适应新的风险-收益目标。
例如,我们可以通过最大化夏普比率(收益与风险的比率)来构建投资组合:
```java
// Java代码:基于夏普比率的贪心策略
public class SharpeRatioStrategy {
private List<Asset> assets; // Asset类包含资产的收益和风险
public SharpeRatioStrategy(List<Asset> assets) {
this.assets = assets;
}
public List<Asset> optimizePortfolio() {
// 按夏普比率降序排列资产
assets.sort((a, b) -> ***pare(b.sharpeRatio(), a.sharpeRatio()));
// 选择夏普比率最高的资产
List<Asset> portfolio = new ArrayList<>();
for (Asset asset : assets) {
if (portfolio.isEmpty() || asset.correlation(portfolio.get(0)) < 1) {
portfolio.add(asset);
}
}
return portfolio;
}
}
```
在此代码中,`optimizePortfolio` 方法通过贪心选择夏普比率最高的资产构建投资组合。注意,这里还考虑了资产之间的相关性,避免了高相关性的资产同时被选中。
### 2.2 贪心算法在投资组合优化中的实现
#### 2.2.1 组合选择的贪心方法
投资组合优化的目标是在满足一定风险约束的情况下最大化收益,或者在满足一定收益目标的情况下最小化风险。贪心算法可以在多种约束条件下应用,用于选择最优的投资组合。
一个典型的投资组合优化问题可以通过以下步骤解决:
1. **定义目标函数**:例如,最大化预期收益或者最小化波动率。
2. **定义约束条件**:例如,个别资产投资比例、投资组合总波动率等。
3. **贪心选择**:按照某种贪心准则选择资产加入组合,如最高预期收益。
4. **局部优化**:调整组合中资产权重,以达到局部最优解。
例如,我们可以使用贪心方法来构建一个风险最小化的投资组合:
```java
// Java代码:贪心方法构建最小风险投资组合
public class MinRiskPortfolio {
private List<Asset> assets; // Asset类包含资产的收益和风险
public MinRiskPortfolio(List<Asset> assets) {
this.assets = assets;
}
public Portfolio buildPortfolio(double totalBudget) {
Portfolio portfolio = new Portfolio();
while (totalBudget > 0) {
Asset bestAsset = null;
double bestReturn = Double.MAX_VALUE;
for (Asset asset : assets) {
double currentReturn = asset.getReturn();
if (currentReturn < bestReturn) {
bestAsset = asset;
bestReturn = currentReturn;
}
}
if (bestAsset != null) {
double allocation = Math.min(totalBudget, bestAsset.getPrice());
portfolio.allocate(bestAsset, allocation);
totalBudget -= allocation;
assets.remove(bestAsset);
}
}
return portfolio;
}
}
```
在此代码示例中,`buildPortfolio` 方法通过贪心地选择预期收益最低的资产,构建了风险最小化的投资组合。
#### 2.2.2 优化策略与案例分析
在实际应用中,贪心算法与其他优化技术结合,可以得到更优的投资组合优化策略。案例分析可以帮助我们了解贪心算法在投资组合优化中是如何应用的。
例如,可以采用贪心算法与遗传算法的混合策略来优化投资组合,其中贪心算法用于局部搜索,而遗传算法用于全局搜索。
### 2.3 贪心算法在金融衍生品定价中的应用
#### 2.3.1 衍生品定价模型与贪心算法
金融衍生品定价是金融工程中一个复杂的领域,但贪心算法可以帮助简化模型的求解过程。在某些情况下,通过贪心选择可以得到一个近似最优的定价结果。
一个典型的贪心策略应用可以是:
1. **定价模型构建**:使用如二叉树定价模型、蒙特卡洛模拟等。
2. **局部最优选择**:在每一步中选择对当前价值最大化的路径。
3. **路径回溯**:从最终状态回溯至初始状态,确定最优路径的策略。
例如,二叉树模型中可以通过贪心选择得到衍生品的近似价值:
```java
// Java代码:使用贪心算法进行期权定价
public class OptionPricingGreedy {
private double upFactor;
private double downFactor;
private double riskFreeRate;
private double expirationTime;
private double underlyingPrice;
private double strikePrice;
private double volatility;
public OptionPricingGreedy(double upFactor, double downFactor, double riskFreeRate,
double expirationTime, double underlyingPrice,
double strikePrice, double volatility) {
this.upFactor = upFactor;
this.downFactor = downFactor;
this.riskFreeRate
```
0
0
相关推荐
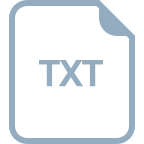
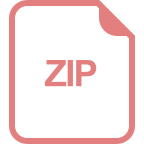
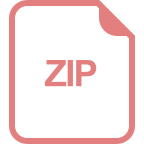
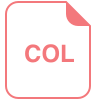
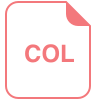
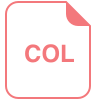
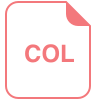
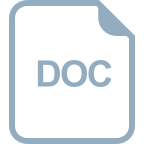
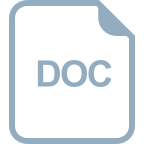