请你使用C语言写一段代码,计算一个不带括号的用字符表示的简单四则运算的值
时间: 2024-11-29 16:38:49 浏览: 10
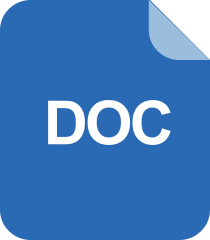
用栈来对一位数的四则运算表达式计算结果
在C语言中,我们可以使用栈来解决这个问题,因为栈是一种LIFO(后进先出)的数据结构,非常适合处理运算符优先级的问题。下面是一个简单的示例,它读取字符串并尝试解析其中的运算符和数字,然后按照正确的顺序执行计算:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAX_STACK_SIZE 50
typedef struct {
int num; // 数字
char op; // 运算符
} Node;
int precedence(char op);
void push(Node* stack, Node node);
double calculate(char* expression);
Node stack[MAX_STACK_SIZE];
int top = -1;
int precedence(char op) {
if (op == '+' || op == '-') return 1;
else if (op == '*' || op == '/') return 2;
return 0;
}
void push(Node* stack, Node node) {
if (top < MAX_STACK_SIZE - 1) {
stack[++top] = node;
}
}
double performOperation(double a, double b, char op) {
switch (op) {
case '+': return a + b;
case '-': return a - b;
case '*': return a * b;
case '/': return a / b;
default: return 0;
}
}
double calculate(char* expression) {
double result = 0;
Node current;
for (int i = 0; expression[i]; ++i) {
if (isdigit(expression[i])) { // 处理数字
current.num = 0;
while (isdigit(expression[i])) {
current.num = current.num * 10 + (expression[i] - '0');
++i;
}
push(&stack, (Node){current.num, '\0'});
} else if (expression[i] == '(') { // 遇到左括号,暂存结果
while (!isspace(expression[i]) && expression[i] != ')') {
calculate(expression + i + 1); // 递归处理内部表达式
}
expression += i; // 跳过右括号
continue;
} else if (expression[i] == ')') { // 遇到右括号,结束当前计算
break;
} else { // 遇到运算符
while (top > 0 && precedence(stack[top].op) >= precedence(expression[i])) {
current = stack[top];
stack[top] = {0, '\0'};
result = performOperation(result, current.num, current.op);
--top;
}
push(&stack, (Node){expression[i], '\0'});
}
}
// 处理剩余的运算符和数字
while (top > 0) {
current = stack[top];
stack[top] = {0, '\0'};
result = performOperation(result, current.num, current.op);
--top;
}
return result;
}
int main() {
char expr[] = "2 + 3 * (4 - 1)";
printf("Result: %.2f\n", calculate(expr));
return 0;
}
```
这个代码会计算给定的简单四则运算表达式的值。注意,这个例子仅适用于没有括号的、连续的运算符和数字,并假设输入字符串有效。如果需要处理更复杂的表达式,如包含括号的情况,可以采用更为复杂的方法,如递归下降解析或使用解析库。
阅读全文
相关推荐
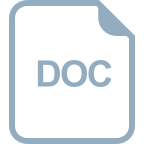
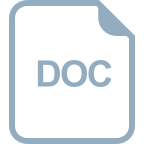

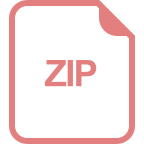
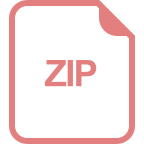
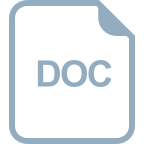
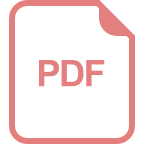
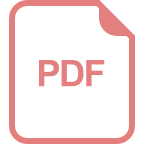
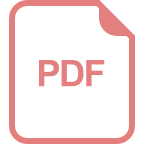
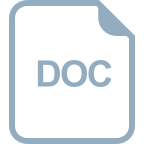
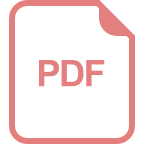
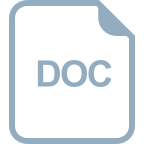
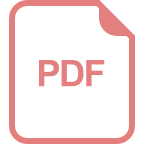
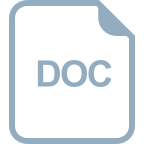
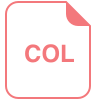
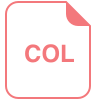
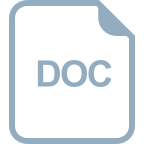
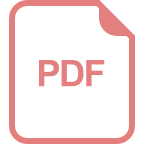
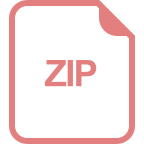