stm32的rtthread 双串口程序
时间: 2023-06-23 21:10:29 浏览: 93
以下是一个基于STM32和RT-Thread操作系统的双串口程序示例:
```c
#include <rtthread.h>
#include <rtdevice.h>
#define USART1_CONFIG \
{ \
.name = "uart1", \
.parent_name = "serial", \
.open_flag = RT_DEVICE_FLAG_RDWR | RT_DEVICE_FLAG_INT_RX | RT_DEVICE_FLAG_INT_TX, \
.close_flag = 0, \
.read = rt_serial_read, \
.write = rt_serial_write, \
.control = rt_serial_control \
}
#define USART2_CONFIG \
{ \
.name = "uart2", \
.parent_name = "serial", \
.open_flag = RT_DEVICE_FLAG_RDWR | RT_DEVICE_FLAG_INT_RX | RT_DEVICE_FLAG_INT_TX, \
.close_flag = 0, \
.read = rt_serial_read, \
.write = rt_serial_write, \
.control = rt_serial_control \
}
static struct rt_serial_configure usart1_config = RT_SERIAL_CONFIG_DEFAULT;
static struct rt_serial_configure usart2_config = RT_SERIAL_CONFIG_DEFAULT;
rt_err_t usart1_rx_ind(rt_device_t dev, rt_size_t size)
{
/* do something when receive interrupt happens */
}
rt_err_t usart2_rx_ind(rt_device_t dev, rt_size_t size)
{
/* do something when receive interrupt happens */
}
void usart1_tx_complete(void *arg)
{
/* do something when transmit complete interrupt happens */
}
void usart2_tx_complete(void *arg)
{
/* do something when transmit complete interrupt happens */
}
int main(void)
{
rt_device_t usart1, usart2;
/* initialize USART1 and USART2 configurations */
usart1_config.baud_rate = 115200;
usart2_config.baud_rate = 9600;
/* register USART1 and USART2 devices */
usart1 = rt_serial_register(&USART1_CONFIG, &usart1_config);
usart2 = rt_serial_register(&USART2_CONFIG, &usart2_config);
/* set interrupt callback functions for USART1 and USART2 receive events */
rt_device_set_rx_indicate(usart1, usart1_rx_ind);
rt_device_set_rx_indicate(usart2, usart2_rx_ind);
/* set interrupt callback functions for USART1 and USART2 transmit events */
rt_device_set_tx_complete(usart1, usart1_tx_complete);
rt_device_set_tx_complete(usart2, usart2_tx_complete);
/* open USART1 and USART2 devices */
rt_device_open(usart1, RT_DEVICE_FLAG_INT_RX | RT_DEVICE_FLAG_INT_TX);
rt_device_open(usart2, RT_DEVICE_FLAG_INT_RX | RT_DEVICE_FLAG_INT_TX);
while (1) {
/* do something */
}
}
```
在这个示例中,首先定义了两个串口设备的配置信息结构体 `usart1_config` 和 `usart2_config`,分别对应 USART1 和 USART2。然后使用 `rt_serial_register` 函数注册这两个串口设备,并设置接收和发送的回调函数。最后使用 `rt_device_open` 函数打开两个串口设备,开始接收和发送数据。在主循环中,可以执行其他任务。
需要注意的是,在此示例中,串口设备的父节点为 "serial",因此需要先在RT-Thread的设备管理器中注册一个名为 "serial" 的设备,才能注册和打开 USART1 和 USART2 等串口设备。
此外,还需要根据实际情况修改串口的波特率、数据位、停止位、校验位等参数。
阅读全文
相关推荐


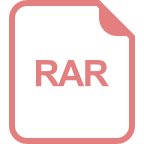
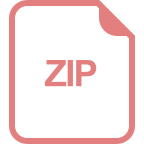
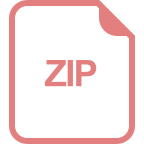
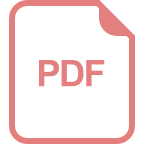
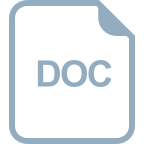
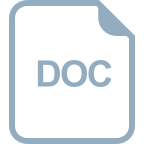
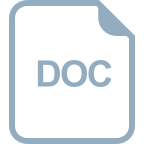

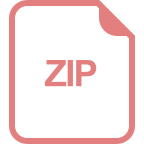
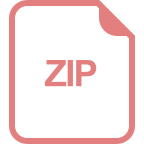
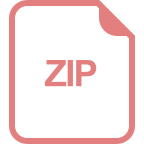
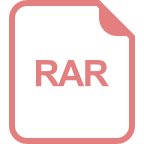
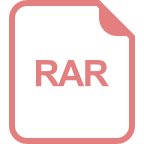
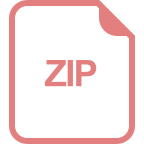