用java实现git对比不同分支的代码增量信息
时间: 2023-12-14 11:37:18 浏览: 33
要实现Git对比不同分支的代码增量信息,可以使用JGit库来操作Git仓库。以下是一个简单的Java代码示例,用于比较两个分支之间的代码差异:
```java
import java.io.IOException;
import java.util.List;
import org.eclipse.jgit.api.Git;
import org.eclipse.jgit.api.errors.GitAPIException;
import org.eclipse.jgit.diff.DiffEntry;
import org.eclipse.jgit.diff.DiffFormatter;
import org.eclipse.jgit.diff.RawTextComparator;
import org.eclipse.jgit.lib.ObjectId;
import org.eclipse.jgit.lib.Repository;
import org.eclipse.jgit.revwalk.RevCommit;
import org.eclipse.jgit.revwalk.RevWalk;
import org.eclipse.jgit.treewalk.CanonicalTreeParser;
import org.eclipse.jgit.treewalk.TreeWalk;
public class GitDiff {
public static void main(String[] args) throws IOException, GitAPIException {
String repositoryPath = "/path/to/your/repository"; // replace with your repository path
String oldBranch = "old-branch"; // replace with the name of the old branch
String newBranch = "new-branch"; // replace with the name of the new branch
try (Repository repository = Git.open(new File(repositoryPath)).getRepository()) {
ObjectId oldHead = repository.resolve(oldBranch + "^{commit}");
ObjectId newHead = repository.resolve(newBranch + "^{commit}");
try (RevWalk revWalk = new RevWalk(repository)) {
RevCommit oldCommit = revWalk.parseCommit(oldHead);
RevCommit newCommit = revWalk.parseCommit(newHead);
CanonicalTreeParser oldTreeParser = new CanonicalTreeParser();
try (ObjectReader oldReader = repository.newObjectReader()) {
oldTreeParser.reset(oldReader, oldCommit.getTree().getId());
}
CanonicalTreeParser newTreeParser = new CanonicalTreeParser();
try (ObjectReader newReader = repository.newObjectReader()) {
newTreeParser.reset(newReader, newCommit.getTree().getId());
}
try (Git git = new Git(repository)) {
List<DiffEntry> diffs = git.diff()
.setOldTree(oldTreeParser)
.setNewTree(newTreeParser)
.call();
for (DiffEntry diff : diffs) {
System.out.println("Diff: " + diff.getChangeType() + " " + diff.getNewPath());
try (DiffFormatter formatter = new DiffFormatter(System.out)) {
formatter.setRepository(repository);
formatter.setDiffComparator(RawTextComparator.DEFAULT);
formatter.format(diff);
}
}
}
}
}
}
}
```
在此示例中,我们使用JGit库打开Git仓库,并使用`Git.open()`方法获取仓库的引用。然后,我们解析旧和新分支的提交ID,并将它们传递给`CanonicalTreeParser`,以便可以比较它们之间的树差异。最后,我们使用`Git.diff()`方法比较树,并使用`DiffFormatter`格式化差异输出。
请注意,这只是一个基本示例,您可能需要根据您的需求进行修改和扩展。
相关推荐
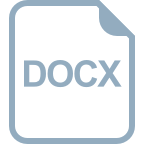













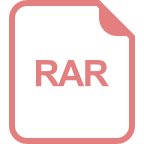