深度学习算法进行人脸检测python代码
时间: 2023-08-19 11:04:03 浏览: 124
使用深度学习算法进行人脸检测的Python代码可以使用OpenCV和Dlib库实现。以下是使用OpenCV进行人脸检测的示例代码:
```python
import cv2
# 加载分类器
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
# 加载图像
img = cv2.imread('test.jpg')
# 转为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 检测人脸
faces = face_cascade.detectMultiScale(gray, 1.3, 5)
# 绘制矩形框
for (x,y,w,h) in faces:
cv2.rectangle(img,(x,y),(x+w,y+h),(255,0,0),2)
# 显示图像
cv2.imshow('img',img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
以上代码中,`haarcascade_frontalface_default.xml`是OpenCV提供的人脸分类器,可以在官方文档中下载。`detectMultiScale`方法可以进行多尺度检测,可以调整检测精度和速度。在检测到人脸后,可以使用`rectangle`方法在图像上绘制矩形框。最后使用`imshow`方法显示图像。
另外,Dlib库也提供了人脸检测的功能,使用方法类似。
相关问题
三种算法实现深度学习python人脸检测代码
以下是三种深度学习算法实现人脸检测的Python代码示例:
1. 基于SSD算法的人脸检测代码:
```python
import cv2
# 加载模型
net = cv2.dnn.readNetFromCaffe('deploy.prototxt', 'res10_300x300_ssd_iter_140000.caffemodel')
# 加载图像
img = cv2.imread('test.jpg')
# 进行预处理
blob = cv2.dnn.blobFromImage(cv2.resize(img, (300, 300)), 1.0, (300, 300), (104.0, 177.0, 123.0))
# 进行预测
net.setInput(blob)
detections = net.forward()
# 绘制矩形框
for i in range(0, detections.shape[2]):
confidence = detections[0, 0, i, 2]
if confidence > 0.5:
box = detections[0, 0, i, 3:7] * np.array([img.shape[1], img.shape[0], img.shape[1], img.shape[0]])
(startX, startY, endX, endY) = box.astype("int")
cv2.rectangle(img, (startX, startY), (endX, endY), (0, 0, 255), 2)
# 显示图像
cv2.imshow('img',img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
2. 基于YOLO算法的人脸检测代码:
```python
import cv2
# 加载模型
net = cv2.dnn.readNet('yolov3-tiny.weights', 'yolov3-tiny.cfg')
# 加载标签
with open('coco.names', 'r') as f:
labels = [line.strip() for line in f.readlines()]
# 加载图像
img = cv2.imread('test.jpg')
# 进行预处理
blob = cv2.dnn.blobFromImage(img, 1/255.0, (416, 416), swapRB=True, crop=False)
# 进行预测
net.setInput(blob)
outputs = net.forward()
# 绘制矩形框
for output in outputs:
for detection in output:
scores = detection[5:]
classId = np.argmax(scores)
confidence = scores[classId]
if confidence > 0.5 and classId == 0:
box = detection[0:4] * np.array([img.shape[1], img.shape[0], img.shape[1], img.shape[0]])
(centerX, centerY, width, height) = box.astype("int")
startX = int(centerX - (width / 2))
startY = int(centerY - (height / 2))
endX = int(centerX + (width / 2))
endY = int(centerY + (height / 2))
cv2.rectangle(img, (startX, startY), (endX, endY), (0, 0, 255), 2)
# 显示图像
cv2.imshow('img',img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
3. 基于MTCNN算法的人脸检测代码:
```python
import cv2
from mtcnn import MTCNN
# 加载模型
detector = MTCNN()
# 加载图像
img = cv2.imread('test.jpg')
# 进行预测
results = detector.detect_faces(img)
# 绘制矩形框
for result in results:
x, y, w, h = result['box']
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 0, 255), 2)
# 显示图像
cv2.imshow('img',img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
以上代码中,分别使用了基于SSD、YOLO和MTCNN算法进行人脸检测。其中,SSD和YOLO都是基于深度学习的目标检测算法,MTCNN则是专门为人脸检测设计的算法。在使用前需要加载对应的模型和标签,并且进行图像预处理和后处理。在预测完成后,可以通过绘制矩形框来标记检测到的人脸。
深度学习人脸识别python代码
深度学习在人脸识别中的应用通常涉及使用卷积神经网络(Convolutional Neural Networks, CNNs)和一些高级技术,如FaceNet、DeepFace或OpenFace。以下是一个简单的概述,不包含完整的代码,但会提供一个基本的概念和步骤:
1. **数据准备**:首先,你需要人脸数据集,如LFW、CelebA或VGGFace等,用于训练模型。数据需要预处理成适合模型输入的格式,如灰度图像和相应的标签。
2. **选择库**:Python中有多个库可以用来进行深度学习,比如TensorFlow, Keras, PyTorch等。选择一个你熟悉的库进行开发。
3. **模型构建**:
- 使用预训练的CNN模型(如VGGFace或者ResNet),作为特征提取器。这些模型已经在大规模数据集上训练过,能够提取人脸的高维特征向量。
- 构建一个自定义层(如FC层)来将特征映射到身份标签。
4. **训练模型**:
- 利用特征提取器提取图像特征,然后训练一个分类器,如softmax层,学习将这些特征与特定的身份关联起来。
- 使用反向传播算法更新模型参数,优化损失函数,如交叉熵损失。
5. **人脸检测**:在实际应用中,可能还需要人脸检测工具,如Dlib或MTCNN,来从图片中准确地找到人脸区域。
6. **评估和部署**:在测试集上评估模型性能,调整超参数,然后将模型部署到应用程序中,比如使用Flask或Django建立Web服务。
以下是部分代码片段示例(简化版):
```python
import tensorflow as tf
from keras.models import Model
from keras.layers import Input, Dense, GlobalAveragePooling2D
# 加载预训练的CNN模型,如VGG16的预去掉最后一层
base_model = VGG16(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
# 添加自定义输出层
x = base_model.output
x = GlobalAveragePooling2D()(x)
predictions = Dense(num_classes, activation='softmax')(x)
# 创建新的模型
model = Model(inputs=base_model.input, outputs=predictions)
# 冻结预训练层以防止更改
for layer in base_model.layers:
layer.trainable = False
# 编译模型
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# 训练模型
model.fit(train_data, train_labels, validation_data=(val_data, val_labels))
```
阅读全文
相关推荐
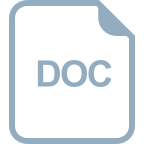
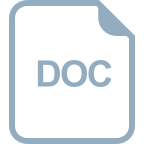
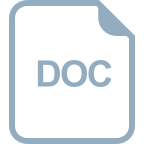
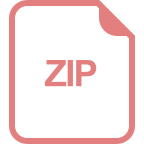
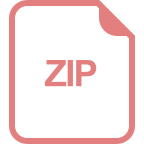
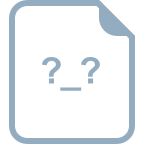
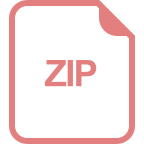
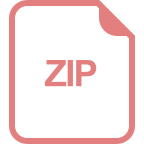
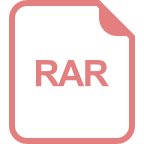
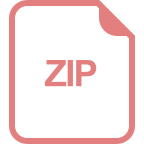
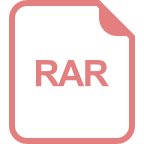
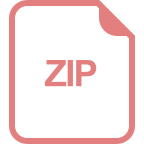
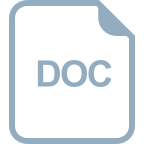
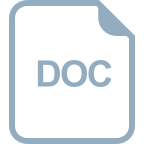


