c#科学计算器用stack
时间: 2023-07-27 19:13:31 浏览: 163
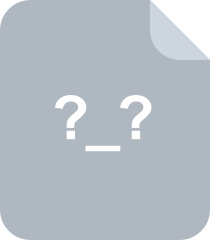
c语言 stack 科学计算器
使用 stack 实现 C# 科学计算器可以很方便地处理中缀表达式,下面是一份简单的实现代码:
```csharp
using System;
using System.Collections.Generic;
namespace ScientificCalculator
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Enter an expression:");
string input = Console.ReadLine();
double result = Calculate(input);
Console.WriteLine("Result: " + result);
}
static double Calculate(string input)
{
Stack<double> numStack = new Stack<double>();
Stack<char> opStack = new Stack<char>();
for (int i = 0; i < input.Length; i++)
{
if (input[i] == ' ')
continue;
if (char.IsDigit(input[i]))
{
string numStr = input[i].ToString();
while (i + 1 < input.Length && (char.IsDigit(input[i + 1]) || input[i + 1] == '.'))
{
numStr += input[i + 1];
i++;
}
double num = double.Parse(numStr);
numStack.Push(num);
}
else if (input[i] == '(')
{
opStack.Push(input[i]);
}
else if (input[i] == ')')
{
while (opStack.Peek() != '(')
{
double num2 = numStack.Pop();
double num1 = numStack.Pop();
char op = opStack.Pop();
double result = ApplyOp(op, num1, num2);
numStack.Push(result);
}
opStack.Pop();
}
else if (IsOperator(input[i]))
{
while (opStack.Count > 0 && Precedence(opStack.Peek()) >= Precedence(input[i]))
{
double num2 = numStack.Pop();
double num1 = numStack.Pop();
char op = opStack.Pop();
double result = ApplyOp(op, num1, num2);
numStack.Push(result);
}
opStack.Push(input[i]);
}
}
while (opStack.Count > 0)
{
double num2 = numStack.Pop();
double num1 = numStack.Pop();
char op = opStack.Pop();
double result = ApplyOp(op, num1, num2);
numStack.Push(result);
}
return numStack.Pop();
}
static bool IsOperator(char c)
{
return c == '+' || c == '-' || c == '*' || c == '/';
}
static int Precedence(char op)
{
if (op == '+' || op == '-')
return 1;
if (op == '*' || op == '/')
return 2;
return 0;
}
static double ApplyOp(char op, double num1, double num2)
{
switch (op)
{
case '+':
return num1 + num2;
case '-':
return num1 - num2;
case '*':
return num1 * num2;
case '/':
if (num2 == 0)
{
throw new DivideByZeroException("Cannot divide by zero");
}
return num1 / num2;
default:
throw new ArgumentException("Invalid operator");
}
}
}
}
```
在上面的代码中,使用了两个 stack 分别存储数字和操作符。具体实现过程如下:
1. 遍历输入表达式,如果当前字符为空格,则忽略;
2. 如果当前字符是数字,则读取完整的数字并将其入数字 stack;
3. 如果当前字符是左括号,则将其入操作符 stack;
4. 如果当前字符是右括号,则不断地弹出操作符 stack 顶端的操作符,直到遇到左括号,并按照顺序计算结果,并将结果入数字 stack;
5. 如果当前字符是操作符,首先判断操作符的优先级,如果当前操作符的优先级小于等于操作符 stack 顶端的操作符,则弹出操作符 stack 顶端的操作符,并按照顺序计算结果,并将结果入数字 stack,然后将当前操作符入操作符 stack;
6. 遍历完成后,不断地弹出操作符 stack 顶端的操作符,并按照顺序计算结果,并将结果入数字 stack,最终数字 stack 中剩下的数字即为表达式的计算结果。
该实现可以处理基本的加减乘除运算以及括号,但是没有实现科学计算器的其他功能,如三角函数、指数函数等。如果需要实现这些功能,需要根据具体需求进行扩展。
阅读全文
相关推荐
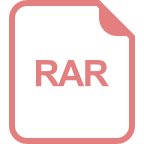


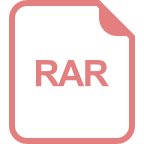
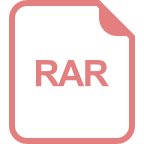
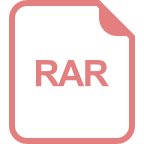
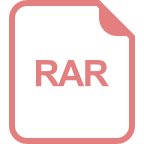
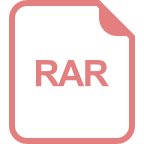
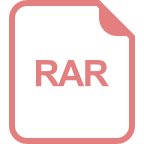
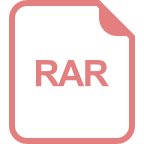
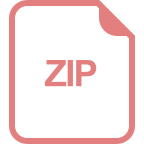
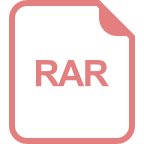
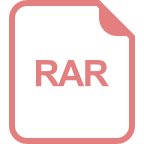
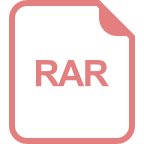
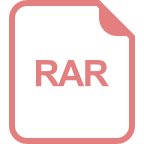
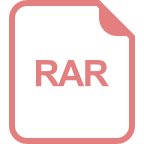