c语言串口发送数据函数
时间: 2023-07-16 11:13:24 浏览: 43
在C语言中,串口发送数据可以使用如下函数:
```c
#include <stdio.h>
#include <string.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
int serialport_write(int fd, const char* str)
{
int len = strlen(str);
int n = write(fd, str, len);
if (n != len) {
return -1;
}
return 0;
}
```
这个函数使用了Linux系统的串口编程库,需要包含头文件`<fcntl.h>`、`<termios.h>`和`<unistd.h>`。其中`fd`参数是串口设备文件的文件描述符,`str`参数是要发送的数据字符串。函数返回0表示发送成功,返回-1表示发送失败。
使用该函数需要先打开串口设备文件,例如:
```c
int fd = open("/dev/ttyUSB0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
printf("Error: Unable to open serial port\n");
return -1;
}
```
上述代码打开了一个名为`/dev/ttyUSB0`的串口设备文件,使用读写模式,并且禁用了控制终端,以免串口被意外中断。如果串口打开失败,返回-1。
完整的示例代码如下:
```c
#include <stdio.h>
#include <string.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
int serialport_write(int fd, const char* str)
{
int len = strlen(str);
int n = write(fd, str, len);
if (n != len) {
return -1;
}
return 0;
}
int main()
{
int fd = open("/dev/ttyUSB0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
printf("Error: Unable to open serial port\n");
return -1;
}
char* msg = "Hello, world!\n";
if (serialport_write(fd, msg) == -1) {
printf("Error: Unable to write to serial port\n");
}
close(fd);
return 0;
}
```
相关推荐
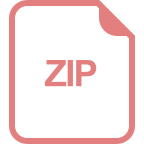
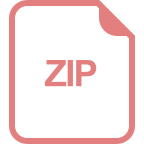














