基于蚁群算法的文件最短路径搜索
时间: 2024-01-20 18:03:54 浏览: 18
基于蚁群算法的文件最短路径搜索是一种启发式算法,它模拟了蚂蚁在搜索食物时释放信息素的行为,通过信息素的积累和挥发,使得蚂蚁能够找到最短路径。在文件最短路径搜索中,我们可以将文件系统中的每个文件夹看作一个城市,文件夹之间的距离根据其在文件系统中的位置关系来计算。下面是基于蚁群算法的文件最短路径搜索的步骤:
1. 定义蚂蚁和路径的数据结构
```java
public class Ant {
private int id;
private int currentCity;
private List<Integer> tabuList;
private List<Integer> allowedCities;
private double tourLength;
}
public class Graph {
private int numOfCities;
private double[][] distances;
}
```
2. 初始化蚂蚁和距离矩阵
```java
public void initAnts() {
// 初始化蚂蚁
for (int i = 0; i < numAnts; i++) {
Ant ant = new Ant();
ant.setId(i);
ant.setTabuList(new ArrayList<Integer>());
ant.setAllowedCities(new ArrayList<Integer>());
for (int j = 0; j < numOfCities; j++) {
ant.getAllowedCities().add(j);
}
ant.setCurrentCity(random.nextInt(numOfCities));
ants.add(ant);
}
// 初始化距离矩阵
distances = new double[numOfCities][numOfCities];
for (int i = 0; i < numOfCities; i++) {
for (int j = 0; j < numOfCities; j++) {
distances[i][j] = graph.getDistances()[i][j];
}
}
}
```
3. 计算蚂蚁在当前城市到其他城市的概率
```java
public double calculateProbability(int i, int j) {
double pheromone = Math.pow(pheromones[i][j], alpha);
double distance = Math.pow(1.0 / distances[i][j], beta);
double sum = 0.0;
for (Integer city : ants.get(i).getAllowedCities()) {
sum += Math.pow(pheromones[i][city], alpha) * Math.pow(1.0 / distances[i][city], beta);
}
return (pheromone * distance) / sum;
}
```
4. 蚂蚁选择下一个城市并更新信息素
```java
public void moveAnts() {
for (Ant ant : ants) {
int currentCity = ant.getCurrentCity();
List<Integer> allowedCities = ant.getAllowedCities();
List<Double> probabilities = new ArrayList<Double>();
for (Integer city : allowedCities) {
probabilities.add(calculateProbability(currentCity, city));
}
int nextCity = rouletteWheelSelection(allowedCities, probabilities);
ant.getTabuList().add(currentCity);
ant.setTourLength(ant.getTourLength() + distances[currentCity][nextCity]);
ant.setCurrentCity(nextCity);
ant.getAllowedCities().remove(new Integer(nextCity));
updatePheromone(currentCity, nextCity, ant.getTourLength());
if (ant.getTabuList().size() == numOfCities) {
ant.setTourLength(ant.getTourLength() + distances[nextCity][ant.getTabuList().get(0)]);
ant.getTabuList().add(nextCity);
}
}
}
```
5. 更新信息素
```java
public void updatePheromone(int i, int j, double tourLength) {
double pheromone = Q / tourLength;
pheromones[i][j] = (1 - rho) * pheromones[i][j] + rho * pheromone;
pheromones[j][i] = pheromones[i][j];
}
```
6. 迭代搜索
```java
public void search() {
// 初始化
initAnts();
// 迭代搜索
for (int i = 0; i < numIterations; i++) {
moveAnts();
updateBest();
updatePheromoneTrail();
reInitAnts();
}
}
```
7. 找到最短路径
```java
public List<Integer> getBestTour() {
Ant bestAnt = null;
for (Ant ant : ants) {
if (bestAnt == null || ant.getTourLength() < bestAnt.getTourLength()) {
bestAnt = ant;
}
}
return bestAnt.getTabuList();
}
```
在实现过程中,还需要使用轮盘赌算法选择下一个城市,更新最优解等。最后,通过调用getBestTour()方法可以得到最短路径。
相关推荐
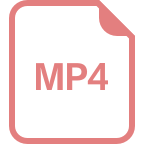
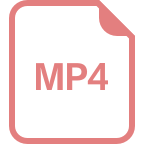
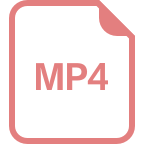







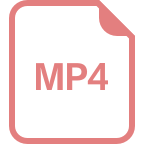
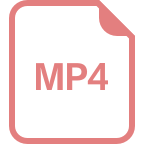
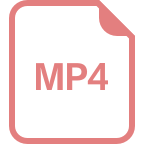
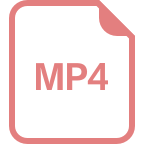
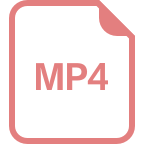
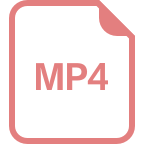
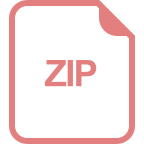
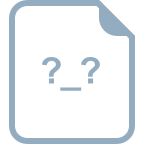