nginx ip hash
时间: 2023-07-18 13:38:14 浏览: 198
在nginx中,IP hash算法是一种基于客户端IP地址的负载均衡方式。具体来说,nginx会根据客户端IP地址的hash值来选择一个后端服务器,这可以确保同一个客户端的请求都会被分配到同一台服务器上,从而保持会话的连续性。
IP hash算法的实现非常简单,只需要在upstream模块中添加ip_hash指令即可,例如:
```
http {
upstream backend {
ip_hash;
server backend1.example.com;
server backend2.example.com;
server backend3.example.com;
}
server {
listen 80;
location / {
proxy_pass http://backend;
}
}
}
```
在这个例子中,nginx会使用IP hash算法将请求分配给名为“backend”的upstream组中的后端服务器。如果同一个客户端发送多个请求,这些请求都会被分配到同一台服务器上。当然,如果有多个客户端使用相同的IP地址,它们也会被分配到同一台服务器上。因此,使用IP hash算法时,需要根据实际情况评估每台服务器的负载情况,以确保负载均衡的效果。
相关问题
nginx刷新iphash记录
Nginx通过IP哈希(ip_hash)模块实现负载均衡,它会将客户端请求的源IP映射到一个固定的后端服务器上,这样可以保持来自同一客户端的请求始终由同一台服务器处理,提供粘性的会话连接。然而,如果服务器列表发生变化,例如添加、删除或重启服务器,IP哈希的映射可能会需要更新。
要刷新Nginx的IP哈希记录,通常不需要直接操作,因为Nginx会在配置文件重启(比如通过`nginx -s reload`命令)后自动更新其内部的哈希表。如果你手动修改了后端服务器的配置,并希望立即生效,可以在`http {}`块下使用`resolver`指令来刷新DNS缓存:
```nginx
http {
resolver <your_resolver_ip>;
# ...
upstream backend {
ip_hash;
server server1.example.com weight=10 max_fails=2 fail_timeout=5s;
server server2.example.com;
# 更改后的其他服务器...
}
}
```
然后运行`nginx -s reload`来应用新的配置。但是请注意,这仅会影响新连接,对已建立的连接不会立即生效。对于实时更改,最好还是依赖于定期的配置更新或服务状态检查。如果有疑问,可以查阅具体的Nginx官方文档。
修改nginx的iphash计算规则
nginx的ip_hash负载均衡算法是基于客户端IP地址进行哈希计算,将相同IP地址的请求分配到同一台后端服务器处理。如果需要修改ip_hash的计算规则,可以通过修改nginx源代码来实现。
具体来说,需要修改src/http/ngx_http_upstream_ip_hash_module.c文件中的ngx_http_upstream_init_ip_hash函数。该函数定义了ip_hash算法的初始化过程,其中有一个ngx_crc32_table_init函数用于初始化crc32哈希表,可以修改该函数中的crc32哈希表来改变ip_hash的计算规则。
下面是一个示例修改,将ip_hash算法中的crc32哈希表替换为murmur3哈希表:
```
#include <ngx_config.h>
#include <ngx_core.h>
#include <ngx_http.h>
static ngx_int_t ngx_http_upstream_init_ip_hash_peer(ngx_http_request_t *r,
ngx_http_upstream_srv_conf_t *us);
static char *ngx_http_upstream_ip_hash(ngx_conf_t *cf, ngx_command_t *cmd,
void *conf);
static void *ngx_http_upstream_ip_hash_create_conf(ngx_conf_t *cf);
static char *ngx_http_upstream_ip_hash_merge_conf(ngx_conf_t *cf,
void *parent, void *child);
static ngx_int_t ngx_http_upstream_init_ip_hash(ngx_conf_t *cf,
ngx_http_upstream_srv_conf_t *us);
static ngx_command_t ngx_http_upstream_ip_hash_commands[] = {
{ ngx_string("ip_hash"),
NGX_HTTP_UPS_CONF|NGX_CONF_NOARGS,
ngx_http_upstream_ip_hash,
0,
0,
NULL },
ngx_null_command
};
static ngx_http_module_t ngx_http_upstream_ip_hash_module_ctx = {
NULL, /* preconfiguration */
NULL, /* postconfiguration */
NULL, /* create main configuration */
NULL, /* init main configuration */
ngx_http_upstream_ip_hash_create_conf, /* create server configuration */
ngx_http_upstream_ip_hash_merge_conf, /* merge server configuration */
NULL, /* create location configuration */
NULL /* merge location configuration */
};
ngx_module_t ngx_http_upstream_ip_hash_module = {
NGX_MODULE_V1,
&ngx_http_upstream_ip_hash_module_ctx, /* module context */
ngx_http_upstream_ip_hash_commands, /* module directives */
NGX_HTTP_MODULE, /* module type */
NULL, /* init master */
NULL, /* init module */
NULL, /* init process */
NULL, /* init thread */
NULL, /* exit thread */
NULL, /* exit process */
NULL, /* exit master */
NGX_MODULE_V1_PADDING
};
static ngx_int_t
ngx_http_upstream_init_ip_hash_peer(ngx_http_request_t *r,
ngx_http_upstream_srv_conf_t *us)
{
ngx_http_upstream_ip_hash_peer_data_t *iphp;
ngx_http_upstream_ip_hash_srv_conf_t *hcf;
ngx_http_upstream_rr_peer_t *peer;
ngx_http_upstream_rr_peers_t *peers;
ngx_uint_t i, n, p;
ngx_http_upstream_state_t *state;
struct sockaddr_in *sin;
u_char buf[NGX_SOCKADDR_STRLEN];
iphp = ngx_pcalloc(r->pool, sizeof(ngx_http_upstream_ip_hash_peer_data_t));
if (iphp == NULL) {
return NGX_ERROR;
}
r->upstream->peer.data = &iphp->rrp;
if (ngx_http_upstream_rr_peer_init(r, us) != NGX_OK) {
return NGX_ERROR;
}
peers = &us->peer->rrp.peers;
n = peers->number;
iphp->hash = ngx_crc32_long(r->connection->sockaddr, r->connection->socklen);
hcf = ngx_http_conf_upstream_srv_conf(us, ngx_http_upstream_ip_hash_module);
p = iphp->hash % peers->total_weight;
iphp->peer = NULL;
for (i = 0; i < n; i++) {
peer = &peers->peer[i];
if (peer->down) {
continue;
}
if ((p -= peer->weight) < 0) {
iphp->peer = peer;
break;
}
}
if (iphp->peer == NULL) {
iphp->peer = &peers->peer[n - 1];
}
sin = (struct sockaddr_in *) iphp->peer->sockaddr;
ngx_log_debug2(NGX_LOG_DEBUG_HTTP, r->connection->log, 0,
"ip_hash peer selected: %ui %V", iphp->hash,
ngx_inet_ntop(AF_INET, &sin->sin_addr, buf, sizeof(buf)));
state = ngx_array_push(&r->upstream_states);
if (state == NULL) {
return NGX_ERROR;
}
state->peer = iphp->peer->peer;
state->sockaddr = iphp->peer->sockaddr;
state->socklen = iphp->peer->socklen;
return NGX_OK;
}
static char *
ngx_http_upstream_ip_hash(ngx_conf_t *cf, ngx_command_t *cmd, void *conf)
{
ngx_http_upstream_srv_conf_t *uscf;
uscf = ngx_http_conf_get_module_srv_conf(cf, ngx_http_upstream_module);
uscf->peer.init_upstream = ngx_http_upstream_init_ip_hash;
uscf->flags = NGX_HTTP_UPSTREAM_CREATE
|NGX_HTTP_UPSTREAM_WEIGHT
|NGX_HTTP_UPSTREAM_MAX_FAILS
|NGX_HTTP_UPSTREAM_FAIL_TIMEOUT
|NGX_HTTP_UPSTREAM_DOWN
|NGX_HTTP_UPSTREAM_BACKUP;
return NGX_CONF_OK;
}
static void *
ngx_http_upstream_ip_hash_create_conf(ngx_conf_t *cf)
{
ngx_http_upstream_ip_hash_srv_conf_t *conf;
conf = ngx_pcalloc(cf->pool, sizeof(ngx_http_upstream_ip_hash_srv_conf_t));
if (conf == NULL) {
return NULL;
}
return conf;
}
static char *
ngx_http_upstream_ip_hash_merge_conf(ngx_conf_t *cf, void *parent, void *child)
{
ngx_http_upstream_ip_hash_srv_conf_t *prev = parent;
ngx_http_upstream_ip_hash_srv_conf_t *conf = child;
ngx_conf_merge_ptr_value(conf->shared, prev->shared, NULL);
return NGX_CONF_OK;
}
```
在上面的示例中,我们定义了一个ngx_http_upstream_init_ip_hash_peer函数,用于初始化ip_hash负载均衡算法。在该函数中,我们使用ngx_crc32_long函数计算客户端IP地址的crc32哈希值,并使用该哈希值来选择后端服务器。我们也可以用其他哈希算法替换该函数中的crc32哈希表,以改变ip_hash的计算规则。最后,我们将ngx_http_upstream_init_ip_hash_peer函数指定为ip_hash负载均衡算法的初始化函数,以便nginx在启动时调用该函数来初始化ip_hash算法。
阅读全文
相关推荐














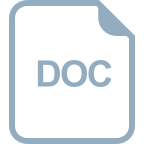
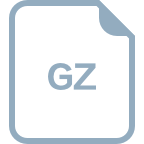