如何使用rospy编写小乌龟走正方形,请给出具体代码
时间: 2023-08-31 18:43:32 浏览: 242
### 回答1:
可以使用以下示例代码实现:import rospy
from geometry_msgs.msg import Twistrospy.init_node('robot_cleaner', anonymous=True)velocity_publisher = rospy.Publisher('/turtle1/cmd_vel', Twist, queue_size=10)vel_msg = Twist()# 前进
vel_msg.linear.x = 2
vel_msg.linear.y = 0
vel_msg.linear.z = 0
vel_msg.angular.x = 0
vel_msg.angular.y = 0
vel_msg.angular.z = 0t0 = rospy.Time.now().to_sec()
current_distance = 0while(current_distance < 10):
velocity_publisher.publish(vel_msg)
t1 = rospy.Time.now().to_sec()
current_distance = 2 * (t1-t0)# 停止小乌龟
vel_msg.linear.x = 0
vel_msg.angular.z = 0
velocity_publisher.publish(vel_msg)# 向左转
vel_msg.linear.x = 0
vel_msg.angular.z = 1
t0 = rospy.Time.now().to_sec()
current_angle = 0while(current_angle < 90):
velocity_publisher.publish(vel_msg)
t1 = rospy.Time.now().to_sec()
current_angle = 1 * (t1-t0)# 停止小乌龟
vel_msg.linear.x = 0
vel_msg.angular.z = 0
velocity_publisher.publish(vel_msg)
### 回答2:
使用rospy编写小乌龟走正方形可以通过控制小乌龟的位姿实现。具体代码如下:
```python
#!/usr/bin/env python
import rospy
from geometry_msgs.msg import Twist
def move_turtle():
rospy.init_node('move_turtle', anonymous=True)
pub = rospy.Publisher('/turtle1/cmd_vel', Twist, queue_size=10)
rate = rospy.Rate(1) # 设置发布频率为1Hz
# 初始化Twist消息
move_cmd = Twist()
move_cmd.linear.x = 1.0 # 设置直线速度为1m/s
move_cmd.angular.z = 0.0 # 设置角速度为0rad/s
side_length = 1 # 正方形边长
duration = 4 # 每条边运动的时间
for i in range(4):
# 向前移动
t0 = rospy.Time.now().to_sec()
while(rospy.Time.now().to_sec() - t0 < duration):
pub.publish(move_cmd)
rate.sleep()
# 停止移动
move_cmd.linear.x = 0.0
pub.publish(move_cmd)
rospy.sleep(1) # 停止运动1秒
# 旋转90度
move_cmd.angular.z = 1.57 # 设置角速度为1.57rad/s
t0 = rospy.Time.now().to_sec()
while(rospy.Time.now().to_sec() - t0 < 1.57):
pub.publish(move_cmd)
rate.sleep()
# 停止旋转
move_cmd.angular.z = 0.0
pub.publish(move_cmd)
rospy.sleep(1) # 停止旋转1秒
rospy.spin()
if __name__ == '__main__':
try:
move_turtle()
except rospy.ROSInterruptException:
pass
```
以上代码通过控制小乌龟的直线速度和角速度,使其按照设定的边长和时间走正方形。首先发布直线速度使小乌龟向前移动,然后改变速度使其停止,再旋转90度,最后再停止旋转。通过循环四次来完成正方形的绘制。
在运行代码之前,需要启动Gazebo仿真器和Turtlesim节点。
### 回答3:
使用rospy编写小乌龟走正方形的具体代码如下:
```python
#!/usr/bin/env python
import rospy
from geometry_msgs.msg import Twist
def move_turtle():
rospy.init_node('move_turtle', anonymous=True)
velocity_publisher = rospy.Publisher('/turtle1/cmd_vel', Twist, queue_size=10)
vel_msg = Twist()
# 设定线速度和角速度
linear_speed = 0.2
angular_speed = 0.2
# 使小乌龟向前移动一定距离
vel_msg.linear.x = linear_speed
vel_msg.linear.y = 0
vel_msg.linear.z = 0
vel_msg.angular.x = 0
vel_msg.angular.y = 0
vel_msg.angular.z = 0
distance = 1 # 设定前进距离
time_to_move = distance/linear_speed
rospy.sleep(1) # 等待节点初始化完成
current_distance = 0
t0 = rospy.Time.now().to_sec()
while(current_distance < distance):
velocity_publisher.publish(vel_msg)
t1=rospy.Time.now().to_sec()
current_distance = linear_speed*(t1-t0)
vel_msg.linear.x = 0
velocity_publisher.publish(vel_msg) # 停止小乌龟
# 使小乌龟旋转
vel_msg.angular.z = angular_speed
t0 = rospy.Time.now().to_sec()
current_angle = 0
target_angle = 90 # 设定旋转角度
while(current_angle < target_angle):
velocity_publisher.publish(vel_msg)
t1=rospy.Time.now().to_sec()
current_angle = angular_speed*(t1-t0)
vel_msg.angular.z = 0
velocity_publisher.publish(vel_msg) # 停止小乌龟
rospy.spin()
if __name__ == '__main__':
try:
move_turtle()
except rospy.ROSInterruptException:
pass
```
以上代码使用rospy包来控制小乌龟机器人在Gazebo仿真环境中以正方形轨迹行走。首先,我们初始化一个ROS节点,并创建一个发布器,该发布器发布速度消息到`/turtle1/cmd_vel`主题上。然后,我们设置小乌龟机器人的线速度和角速度。接着,我们使小乌龟机器人向前移动一定距离,然后停止。然后,我们使小乌龟机器人旋转一定角度,然后停止。最后,我们使ROS节点保持运行,以接收来自其他节点的消息。
阅读全文
相关推荐
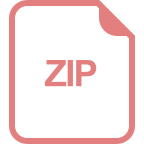
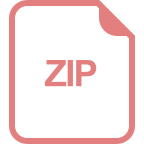

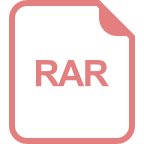
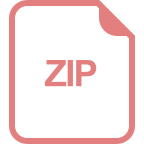
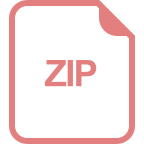
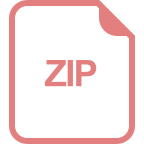
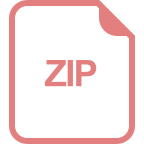
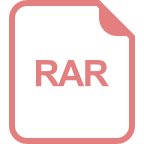
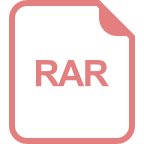
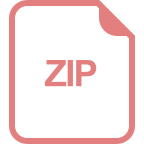



