@RabbitListener注解中bindings作用
时间: 2023-12-08 22:50:50 浏览: 75
`@RabbitListener`注解中的`bindings`属性用于指定消费者监听的队列和交换机及其绑定关系。它是一个数组,每个元素都代表一个绑定关系。
例如,假设我们有一个名为`myQueue`的队列和一个名为`myExchange`的交换机,我们可以使用以下代码将它们绑定在一起:
```java
@RabbitListener(bindings = @QueueBinding(
value = @Queue(value = "myQueue"),
exchange = @Exchange(value = "myExchange", type = ExchangeTypes.TOPIC),
key = "myRoutingKey"
))
public void handleMessage(String message) {
// 处理消息
}
```
在上面的代码中,我们将`myQueue`队列和`myExchange`交换机通过`myRoutingKey`路由键进行绑定,并且使用`@RabbitListener`注解指定了这个绑定关系,当有消息被发送到`myQueue`队列时,`handleMessage`方法会被自动调用来处理消息。
相关问题
RabbitMQ中@RabbitListener注解使用pull模式该怎么设置
RabbitMQ的@RabbitListener注解默认使用push模式,但也可以设置为pull模式。在使用pull模式时,需要将containerType属性设置为SimpleMessageListenerContainer,并配置acknowledgeMode为MANUAL。
示例代码如下:
```java
@RabbitListener(
id = "myListener",
containerFactory = "myContainerFactory",
bindings = @QueueBinding(
value = @Queue(value = "myQueue"),
exchange = @Exchange(value = "myExchange", type = "topic"),
key = "myKey"
),
containerType = SimpleMessageListenerContainer.class,
acknowledgeMode = AcknowledgeMode.MANUAL
)
public void handleMessage(Message message, Channel channel) throws IOException {
// 处理消息
channel.basicAck(message.getMessageProperties().getDeliveryTag(), false);
}
```
其中,myContainerFactory是自定义的SimpleRabbitListenerContainerFactory实例。在该实例中,可以设置prefetchCount属性,以控制每次从消息队列拉取的消息数量。例如:
```java
@Bean
public SimpleRabbitListenerContainerFactory myContainerFactory(ConnectionFactory connectionFactory) {
SimpleRabbitListenerContainerFactory factory = new SimpleRabbitListenerContainerFactory();
factory.setConnectionFactory(connectionFactory);
factory.setAcknowledgeMode(AcknowledgeMode.MANUAL);
factory.setPrefetchCount(10); // 每次从队列中拉取10条消息
return factory;
}
```
@RabbitListener的使用详解
@RabbitListener 是 Spring AMQP 框架中用于监听 RabbitMQ 队列消息的注解。下面是关于 @RabbitListener 使用的详解:
1. 引入依赖:首先,你需要在项目的 pom.xml 文件中添加 Spring AMQP 的依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-amqp</artifactId>
</dependency>
```
2. 创建 RabbitMQ 配置:接下来,你需要在 Spring Boot 项目中创建一个 RabbitMQ 的配置类,用于配置 RabbitMQ 连接信息和相关的队列、交换机等信息。你可以通过 @Configuration 注解来标记这个类,并使用 @Bean 注解来创建 RabbitMQ 的连接工厂和其他必要的组件。
```java
@Configuration
public class RabbitMQConfig {
@Value("${spring.rabbitmq.host}")
private String host;
@Value("${spring.rabbitmq.port}")
private int port;
@Value("${spring.rabbitmq.username}")
private String username;
@Value("${spring.rabbitmq.password}")
private String password;
@Bean
public ConnectionFactory connectionFactory() {
CachingConnectionFactory connectionFactory = new CachingConnectionFactory();
connectionFactory.setHost(host);
connectionFactory.setPort(port);
connectionFactory.setUsername(username);
connectionFactory.setPassword(password);
return connectionFactory;
}
// 其他必要的组件配置...
}
```
3. 创建消息监听器:使用 @RabbitListener 注解来标记一个方法作为消息监听器。你可以在该方法上添加 @QueueBinding 注解来绑定队列和交换机,并指定相关的路由键。
```java
@Component
public class MessageListener {
@RabbitListener(bindings = @QueueBinding(
value = @Queue(value = "myQueue", durable = "true"),
exchange = @Exchange(value = "myExchange", type = ExchangeTypes.TOPIC),
key = "myRoutingKey"
))
public void handleMessage(String message) {
// 处理接收到的消息
System.out.println("Received message: " + message);
}
}
```
在上面的例子中,我们将一个名为 "myQueue" 的队列绑定到名为 "myExchange" 的交换机上,使用 "myRoutingKey" 作为路由键。当有消息到达队列时,会调用 handleMessage 方法进行处理。
4. 启用 RabbitMQ 监听:在启动类上添加 @EnableRabbit 注解,启用 RabbitMQ 的监听功能。
```java
@SpringBootApplication
@EnableRabbit
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
现在,当有消息发送到名为 "myQueue" 的队列时,@RabbitListener 注解所标记的方法将会被触发,并处理接收到的消息。
这就是 @RabbitListener 的使用详解。希望对你有所帮助!如果你还有其他问题,请继续提问。
阅读全文
相关推荐
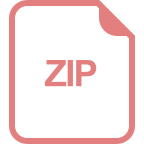
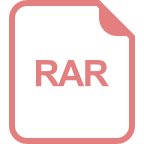
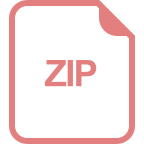



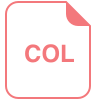
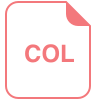
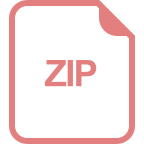