kernel.printk = 5 4 1 7
时间: 2024-05-18 19:16:17 浏览: 10
这是一个 Linux 内核的配置参数,用于设置内核消息的输出级别。具体来说,这里的数字表示四个级别:
- 5:打印所有的内核信息,包括调试信息、信息、警告和错误。
- 4:打印信息、警告和错误,但不包括调试信息。
- 1:只打印紧急情况下的错误信息。
- 7:打印所有内核信息,包括调试信息、信息、警告和错误,并将它们发送到控制台和系统日志。
通常情况下,我们可以将这个参数设置为默认值,即 7,以便获取尽可能多的内核信息,方便进行故障排查和调试。
相关问题
echo 4 4 1 7 >/proc/sys/kernel/printk的作用
该命令的作用是将日志级别设置为指定的值。具体来说,每个数字代表一个级别,数字越高表示级别越严重,例如:
- 0:Emergency messages(紧急消息)
- 1:Alert messages(警戒消息)
- 2:Critical messages(严重消息)
- 3:Error messages(错误消息)
- 4:Warning messages(警告消息)
- 5:Notice messages(通知消息)
- 6:Informational messages(信息消息)
- 7:Debugging messages(调试消息)
该命令中,四个数字分别对应控制台、日志缓冲区、同步文件系统日志和异步文件系统日志的日志级别。因此,命令的含义是将控制台和日志缓冲区的日志级别设置为4(Warning messages),将同步文件系统日志的日志级别设置为1(Alert messages),将异步文件系统日志的日志级别设置为7(Debugging messages)。
这个命令 echo 0 4 1 7 > /proc/sys/kernel/printk 解释
这个命令是将四个数字(0、4、1、7)写入到Linux内核的/proc/sys/kernel/printk文件中。
在Linux系统中,/proc/sys/目录下的文件都是虚拟文件,它们提供了一些内核参数的控制和调整。/proc/sys/kernel/printk文件是一个用于设置内核消息级别的文件。
这个命令中的四个数字分别表示:
- 第一个数字0表示控制台的日志级别,表示打印所有级别的日志信息。
- 第二个数字4表示默认日志级别,表示只打印级别为警告(warning)、错误(error)、紧急(emergency)的日志信息。
- 第三个数字1表示控制台日志级别的持久化,表示在重启后仍然使用第一个数字0设置的日志级别。
- 第四个数字7表示默认日志级别的持久化,表示在重启后仍然使用第二个数字4设置的日志级别。
这个命令的作用是设置内核日志级别,以便在系统运行时记录相关信息,方便调试和故障排查。
相关推荐
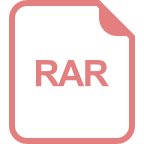
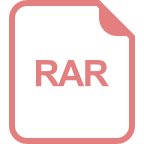
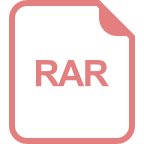













