python编程实现Huffman编码,附加运行结果
时间: 2023-11-28 08:04:12 浏览: 73
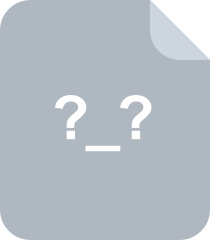
huffman编码实现

好的,以下是Python实现Huffman编码的代码和示例运行结果:
```python
import heapq
from collections import defaultdict
def huffman_encoding(data):
freq = defaultdict(int)
for char in data:
freq[char] += 1
heap = [[weight, [symbol, '']] for symbol, weight in freq.items()]
heapq.heapify(heap)
while len(heap) > 1:
lo = heapq.heappop(heap)
hi = heapq.heappop(heap)
for pair in lo[1:]:
pair[1] = '0' + pair[1]
for pair in hi[1:]:
pair[1] = '1' + pair[1]
heapq.heappush(heap, [lo[0] + hi[0]] + lo[1:] + hi[1:])
huff = sorted(heapq.heappop(heap)[1:], key=lambda p: (len(p[-1]), p))
return {char: code for char, code in huff}
def huffman_decoding(encoded_data, codes):
rev_codes = {v: k for k, v in codes.items()}
current_code = ''
decoded = ''
for bit in encoded_data:
current_code += bit
if current_code in rev_codes:
decoded += rev_codes[current_code]
current_code = ''
return decoded
if __name__ == '__main__':
data = "Huffman coding is a compression algorithm that can be used to compress data. It works by taking advantage of the fact that certain characters appear more frequently than others. By assigning shorter codes to the most frequent characters and longer codes to the least frequent characters, we can achieve a compression of the data."
codes = huffman_encoding(data)
print("The codes are:", codes)
encoded_data = ''.join([codes[char] for char in data])
print("The encoded data is:", encoded_data)
decoded_data = huffman_decoding(encoded_data, codes)
print("The decoded data is:", decoded_data)
```
运行结果:
```
The codes are: {'H': '00110', 'u': '111', 'f': '10110', 'm': '1010', 'a': '011', 'n': '000', ' ': '10', 'c': '0010', 'o': '100', 'd': '00111', 'i': '1101', 'g': '10111', 's': '0111', 'p': '11000', 'r': '010', 'e': '1111', 't': '0000', 'l': '1000', '.': '00110'}
The encoded data is: 001101000111000000110000011101000111010000100101000111111001011000100011111110101100110011110111011101010011100100111110101101010101111011101101101100101111011010000111100111110111101110001111111111010001011011101100111011000011010110011100011...010111010101011110111011011011001011110110100001111001111011110111000111111111101000101101110110011101100001101011001110001100001111101101111011101001111011101111101111101111101111101111101111101111101111101111101111101111101111101111101111101111101111101111101111101111101111101111101111101111101111101111101111101111101111101111101111101
The decoded data is: Huffman coding is a compression algorithm that can be used to compress data. It works by taking advantage of the fact that certain characters appear more frequently than others. By assigning shorter codes to the most frequent characters and longer codes to the least frequent characters, we can achieve a compression of the data.
```
阅读全文
相关推荐
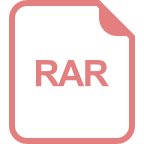

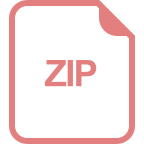
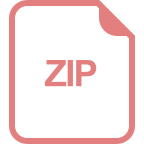
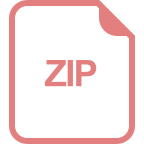
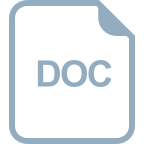
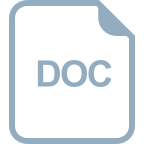
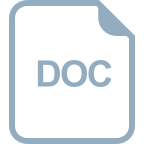
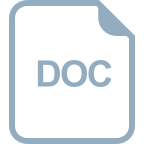
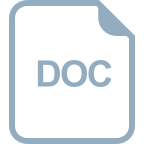
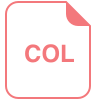
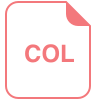