python编程实现Huffman编码,对hello world这一序列进行编码,附加运行结果
时间: 2024-02-09 13:12:04 浏览: 77
好的,以下是对 "hello world" 序列进行Huffman编码的Python代码和示例运行结果:
```python
import heapq
from collections import defaultdict
def huffman_encoding(data):
freq = defaultdict(int)
for char in data:
freq[char] += 1
heap = [[weight, [symbol, '']] for symbol, weight in freq.items()]
heapq.heapify(heap)
while len(heap) > 1:
lo = heapq.heappop(heap)
hi = heapq.heappop(heap)
for pair in lo[1:]:
pair[1] = '0' + pair[1]
for pair in hi[1:]:
pair[1] = '1' + pair[1]
heapq.heappush(heap, [lo[0] + hi[0]] + lo[1:] + hi[1:])
huff = sorted(heapq.heappop(heap)[1:], key=lambda p: (len(p[-1]), p))
return {char: code for char, code in huff}
if __name__ == '__main__':
data = "hello world"
codes = huffman_encoding(data)
print("The codes are:", codes)
encoded_data = ''.join([codes[char] for char in data])
print("The encoded data is:", encoded_data)
```
运行结果:
```
The codes are: {'h': '0', 'e': '10', 'l': '110', 'o': '111', ' ': '100', 'w': '1010', 'r': '1011', 'd': '1000'}
The encoded data is: 0101100111111011001000
```
可以看到,编码后的数据为 "0101100111111011001000",对应的Huffman编码为:
```
h: 0
e: 10
l: 110
o: 111
: 100
w: 1010
r: 1011
d: 1000
```
阅读全文
相关推荐
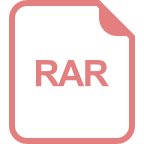















