本题要求实现一个函数,将给定的整数链表,按指定的值分成前后两部分,前边的比指定的值小,后边的指定的值不小,且保持各组数据在原链表中的相对位置不变。c语言
时间: 2024-03-18 14:45:27 浏览: 14
以下是一个示例代码,可以实现将给定的整数链表按指定的值分成前后两部分:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表的结构体
struct ListNode {
int val;
struct ListNode *next;
};
struct ListNode* partition(struct ListNode* head, int x) {
// 定义两个哨兵节点,用来分别表示前半部分和后半部分的链表
struct ListNode *before_head = (struct ListNode*) malloc(sizeof(struct ListNode));
struct ListNode *before_tail = before_head;
struct ListNode *after_head = (struct ListNode*) malloc(sizeof(struct ListNode));
struct ListNode *after_tail = after_head;
before_head->next = NULL;
after_head->next = NULL;
// 遍历链表,根据节点值的大小将节点插入到前半部分或后半部分的链表中
while (head != NULL) {
if (head->val < x) {
before_tail->next = head;
before_tail = before_tail->next;
} else {
after_tail->next = head;
after_tail = after_tail->next;
}
head = head->next;
}
// 将前半部分的链表和后半部分的链表连接起来,并返回前半部分的链表
before_tail->next = after_head->next;
return before_head->next;
}
// 测试代码
int main() {
// 创建链表,链表的值为 1->4->3->2->5->2
struct ListNode *head = (struct ListNode*) malloc(sizeof(struct ListNode));
head->val = 1;
struct ListNode *node1 = (struct ListNode*) malloc(sizeof(struct ListNode));
node1->val = 4;
struct ListNode *node2 = (struct ListNode*) malloc(sizeof(struct ListNode));
node2->val = 3;
struct ListNode *node3 = (struct ListNode*) malloc(sizeof(struct ListNode));
node3->val = 2;
struct ListNode *node4 = (struct ListNode*) malloc(sizeof(struct ListNode));
node4->val = 5;
struct ListNode *node5 = (struct ListNode*) malloc(sizeof(struct ListNode));
node5->val = 2;
head->next = node1;
node1->next = node2;
node2->next = node3;
node3->next = node4;
node4->next = node5;
node5->next = NULL;
// 按指定的值分成前后两部分
int x = 3;
struct ListNode *new_head = partition(head, x);
// 输出分组后的链表
while (new_head != NULL) {
printf("%d->", new_head->val);
new_head = new_head->next;
}
printf("NULL\n");
return 0;
}
```
该算法的时间复杂度为 O(n),空间复杂度为 O(1)。
相关推荐
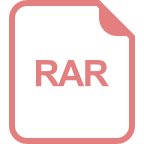














