修改#include<iostream> #include<algorithm> using namespace std; class Node { public: int val; Node* next; Node() { this->next=NULL; } }; class list { public: Node *head; list() //构造函数 { // head=new Node(); // head->next=NULL; } list nextL (Node*p) //以下一个节点为开始的新链表 { list l; l.head->val=p->val; l.head->next=p->next; return l; } }; Node* createList(int n) //创建长度为n的链表(尾插法) { if(n==0) return NULL; Node*p=new Node; cin>>p->val; p->next=createList(n-1); return p; } void Question7(); int maxNode(list l); int minNode(list l); int main() { Question7(); } void Question7() { list l; cout<<"输入长度为10的链表的值:"<<endl; Node*p=createList(10); //创建链表 l.head=p; cout<<"最大值"<<maxNode(l)<<endl; cout<<"最小值"<<minNode(l)<<endl; } int maxNode(list l) { Node*p=l.head; if(p->next==NULL) //走到最后返回这个点的值 return p->val; return max(p->val,maxNode(l.nextL(p->next))); //返回这个点及其往后的最大值 } int minNode(list l) { Node*p=l.head; if(p->next==NULL) //走到最后返回这个点的值 return p->val; return min(p->val,minNode(l.nextL(p->next))); //返回这个点及其往后的最小值 }
时间: 2024-02-04 21:02:29 浏览: 21
这是修改后的代码,主要是增加了list类的成员函数nextL用于创建下一个以某个节点为开始的新链表,并且可以在l.head未初始化的情况下修改它的值:
```c++
#include<iostream>
#include<algorithm>
using namespace std;
class Node {
public:
int val;
Node* next;
Node() {
this->next=NULL;
}
};
class list {
public:
Node *head;
list() {
head=new Node();
// head->next=NULL;
}
list nextL (Node*p) {
list l;
l.head=p;
return l;
}
};
Node* createList(int n) {
if(n==0) return NULL;
Node*p=new Node;
cin>>p->val;
p->next=createList(n-1);
return p;
}
void Question7();
int maxNode(list l);
int minNode(list l);
int main() {
Question7();
}
void Question7() {
list l;
cout<<"输入长度为10的链表的值:"<<endl;
Node*p=createList(10);
l.head=p;
cout<<"最大值:"<<maxNode(l)<<endl;
cout<<"最小值:"<<minNode(l)<<endl;
}
int maxNode(list l) {
Node*p=l.head;
if(p->next==NULL)
return p->val;
return max(p->val,maxNode(l.nextL(p->next).head));
}
int minNode(list l) {
Node*p=l.head;
if(p->next==NULL)
return p->val;
return min(p->val,minNode(l.nextL(p->next).head));
}
```
这个程序创建了一个链表,然后使用递归的方式查找链表中的最大值和最小值。在list类中,nextL函数返回以某个节点为开始的新链表。在查找最大值和最小值时,如果已经到达链表的末尾,则返回当前节点的值。否则,递归调用nextL函数并传入下一个节点,继续查找下一个节点的值,最后返回当前节点和后面的所有节点的最大值或最小值。
相关推荐
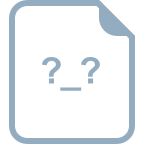















