linux websocket 服务器程序
时间: 2023-11-21 20:54:36 浏览: 235
websocketd是一个在Linux上运行的WebSocket守护程序,它可以处理WebSocket连接并启动程序以处理WebSocket请求。如果您可以从命令行运行程序,则可以编写WebSocket端点。只需从stdin中读取传入的文本,处理它并将结果写回stdout即可。此外,还有其他一些可用于Linux的WebSocket服务器程序,例如Node.js的ws模块和C++的WebSocket++库等。这些程序都可以在Linux上运行,并提供WebSocket服务器功能。
相关问题
Linux C++中实现WebSocket服务器端转发行情数据
要在Linux C++中实现WebSocket服务器端转发行情数据,可以使用以下步骤:
1. 使用第三方C++库,如libwebsockets或uWebSockets等,来实现WebSocket服务器端。
2. 在服务器端编写代码,以便将行情数据发送到WebSocket客户端。可以使用网络库,如boost::asio或ACE等,来管理网络连接和数据传输。
3. 在WebSocket服务器端中,使用JSON格式将行情数据打包并发送给客户端。可以使用第三方JSON库,如RapidJSON或nlohmann/json等。
4. 为了便于管理和扩展,可以将WebSocket服务器端和行情数据转发逻辑分离到不同的模块中。
下面是一个简单的示例代码,展示如何使用libwebsockets库实现WebSocket服务器端转发行情数据:
```c++
#include <libwebsockets.h>
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 行情数据结构体
struct Quote {
string symbol;
double price;
};
// 行情数据源
class QuoteSource {
public:
void addQuote(const Quote& quote) {
quotes.push_back(quote);
}
const vector<Quote>& getQuotes() const {
return quotes;
}
private:
vector<Quote> quotes;
};
// WebSocket服务器端
class WebSocketServer {
public:
WebSocketServer(int port) : port(port), context(NULL), listen_socket(NULL), quote_source(NULL) {}
~WebSocketServer() {
if (listen_socket) {
libwebsocket_close_and_free_session(context, listen_socket, LWS_CLOSE_STATUS_GOINGAWAY);
}
if (context) {
libwebsocket_context_destroy(context);
}
}
void setQuoteSource(QuoteSource* qs) {
quote_source = qs;
}
bool start() {
// 创建WebSocket协议上下文
struct libwebsocket_protocols protocols[] = {
{ "http-only", callback_http, 0 },
{ "quote", callback_quote, sizeof(struct Quote) },
{ NULL, NULL, 0 }
};
struct libwebsocket_context_creation_info info = {
.port = port,
.protocols = protocols,
.gid = -1,
.uid = -1
};
context = libwebsocket_create_context(&info);
if (!context) {
cerr << "Failed to create WebSocket context" << endl;
return false;
}
// 监听WebSocket连接
listen_socket = libwebsocket_create_server(context, 0, protocols, NULL, NULL, NULL, NULL, -1, -1);
if (!listen_socket) {
cerr << "Failed to create WebSocket server" << endl;
libwebsocket_context_destroy(context);
return false;
}
cout << "WebSocket server started on port " << port << endl;
return true;
}
private:
// HTTP回调函数
static int callback_http(struct libwebsocket_context* context, struct libwebsocket* wsi, enum libwebsocket_callback_reasons reason, void* user, void* in, size_t len) {
return 0;
}
// 行情数据回调函数
static int callback_quote(struct libwebsocket_context* context, struct libwebsocket* wsi, enum libwebsocket_callback_reasons reason, void* user, void* in, size_t len) {
switch (reason) {
case LWS_CALLBACK_ESTABLISHED:
// 新客户端连接
cout << "WebSocket client connected" << endl;
break;
case LWS_CALLBACK_SERVER_WRITEABLE:
// 发送行情数据到客户端
if (quote_source) {
const vector<Quote>& quotes = quote_source->getQuotes();
for (size_t i = 0; i < quotes.size(); ++i) {
libwebsocket_write(wsi, (unsigned char*)"es[i], sizeof(Quote), LWS_WRITE_BINARY);
}
}
break;
case LWS_CALLBACK_CLOSED:
// 客户端断开连接
cout << "WebSocket client disconnected" << endl;
break;
default:
break;
}
return 0;
}
private:
int port;
struct libwebsocket_context* context;
struct libwebsocket* listen_socket;
QuoteSource* quote_source;
};
int main() {
// 创建WebSocket服务器
WebSocketServer server(8080);
// 创建行情数据源
QuoteSource quote_source;
// 添加行情数据
quote_source.addQuote({ "AAPL", 123.45 });
quote_source.addQuote({ "GOOGL", 234.56 });
// 设置行情数据源
server.setQuoteSource("e_source);
// 启动WebSocket服务器
if (!server.start()) {
return 1;
}
// 运行事件循环
while (true) {
libwebsocket_service(server.getContext(), 50);
}
return 0;
}
```
在上面的示例代码中,我们使用libwebsockets库创建了一个WebSocket服务器,并且在服务器端实现了一个行情数据源。在行情数据源中,我们添加了两个行情数据(AAPL和GOOGL),然后将行情数据源设置为WebSocket服务器的属性。在回调函数中,我们使用libwebsocket_write函数将行情数据发送到WebSocket客户端。
当我们运行这个示例程序时,它将启动一个WebSocket服务器,监听8080端口。如果我们使用浏览器或其他WebSocket客户端连接到这个服务器,它将发送行情数据到客户端。
阅读全文
相关推荐
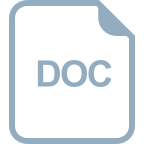
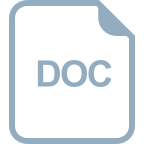
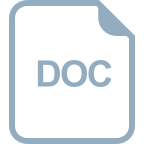
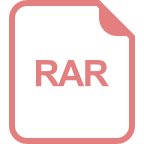
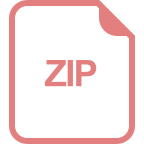
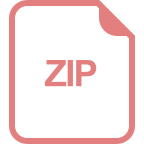
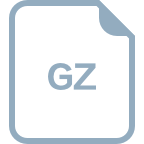
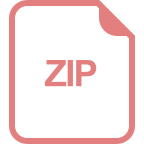
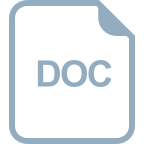
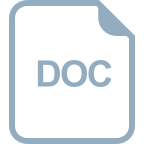
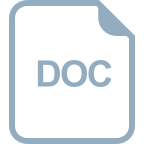
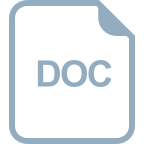
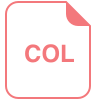
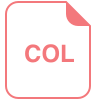
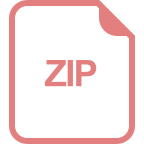
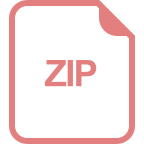
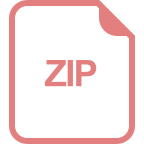
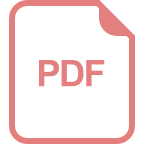