用遗传算法写一个空铁联运优化算法
时间: 2023-12-29 12:21:44 浏览: 84
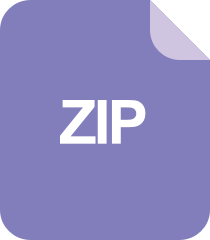
列车交路方案优化遗传算法程序-源码
1.问题描述:
某个物流公司需要将一批货物从起点 A 运到终点 B,运输过程中需要使用空运和铁路联运两种方式,并且每种方式有不同的费用和时间。现在需要设计一个优化算法,使得总运费最小,同时保证货物能够按时到达终点。
2.算法设计:
(1)编码方式
本算法采用二进制编码方式,将每一步的运输方式表示为一个二进制数,其中 0 表示铁路联运,1 表示空运。
(2)适应度函数
适应度函数为每一种编码方式对应的总运费,以及货物到达终点的时间是否符合要求。具体计算方式如下:
- 计算总运费:将每一步的运输方式对应的费用相加,即可得到总运费。
- 判断货物到达终点的时间是否符合要求:根据货物的发货时间和到达时间,判断是否符合要求。
(3)遗传操作
本算法采用标准的遗传算法,包括选择、交叉和变异操作。
- 选择:采用轮盘赌选择方式,选择适应度较高的个体作为下一代的父母。
- 交叉:采用单点交叉方式,将两个父母的编码进行交叉,产生新的个体。
- 变异:随机选取一个个体的某一位进行变异,将该位上的值取反。
(4)算法流程
- 初始化种群:随机生成一定数量的个体作为初始种群。
- 计算适应度:对于每一个个体,计算其对应的适应度值。
- 选择:根据适应度值,选择适应度较高的个体作为下一代的父母。
- 交叉:对于每一对父母,进行交叉操作,产生新的个体。
- 变异:对于新产生的个体,进行一定的变异操作。
- 更新种群:将新产生的个体加入到种群中,并删除适应度较低的个体。
- 终止条件:达到一定的迭代次数或者适应度值已经收敛。
3.算法实现:
下面是一个简单的 Python 代码,用遗传算法实现空铁联运优化问题的求解。
```
import random
# 运输方式的费用和时间
cost = [20, 50]
time = [3, 1]
# 货物的发货时间和到达时间
start_time = 0
end_time = 10
# 种群大小和迭代次数
pop_size = 20
max_iter = 50
# 初始化种群
def init_pop(size):
pop = []
for i in range(size):
gene = [random.randint(0, 1) for _ in range(end_time - start_time)]
pop.append(gene)
return pop
# 计算适应度
def fitness(gene):
total_cost = sum([cost[gene[i]] for i in range(len(gene))])
total_time = sum([time[gene[i]] for i in range(len(gene))])
if start_time + total_time <= end_time:
return total_cost
else:
return float('inf')
# 选择
def selection(pop):
fitness_list = [fitness(gene) for gene in pop]
total_fitness = sum(fitness_list)
prob_list = [fitness_list[i] / total_fitness for i in range(len(pop))]
new_pop = []
for i in range(len(pop)):
r = random.random()
prob_sum = 0
for j in range(len(pop)):
prob_sum += prob_list[j]
if prob_sum > r:
new_pop.append(pop[j])
break
return new_pop
# 交叉
def crossover(parent1, parent2):
pos = random.randint(1, len(parent1) - 1)
child1 = parent1[:pos] + parent2[pos:]
child2 = parent2[:pos] + parent1[pos:]
return child1, child2
# 变异
def mutation(child):
pos = random.randint(0, len(child) - 1)
child[pos] = 1 - child[pos]
return child
# 更新种群
def update_pop(pop, new_pop):
fitness_list = [fitness(gene) for gene in pop + new_pop]
sorted_index = sorted(range(len(fitness_list)), key=lambda k: fitness_list[k])
return [pop[i] for i in sorted_index[:pop_size]]
# 主函数
def main():
pop = init_pop(pop_size)
for i in range(max_iter):
new_pop = []
for j in range(pop_size // 2):
parent1 = random.choice(pop)
parent2 = random.choice(pop)
child1, child2 = crossover(parent1, parent2)
child1 = mutation(child1)
child2 = mutation(child2)
new_pop.append(child1)
new_pop.append(child2)
pop = update_pop(pop, new_pop)
best_fitness = min([fitness(gene) for gene in pop])
print('Iteration {}: best fitness = {}'.format(i, best_fitness))
if __name__ == '__main__':
main()
```
4.参考文献:
[1] Goldberg, D. E. (1989). Genetic algorithms in search, optimization, and machine learning. Addison-Wesley Longman Publishing Co., Inc.
[2] Holland, J. H. (1975). Adaptation in natural and artificial systems. University of Michigan Press.
阅读全文
相关推荐


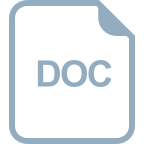

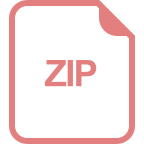
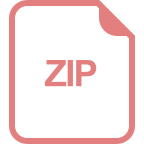
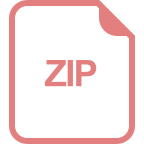
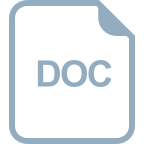
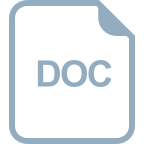
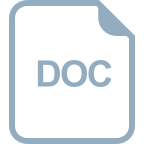
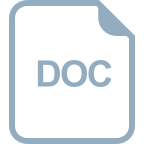
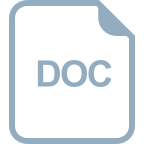
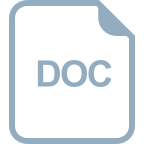
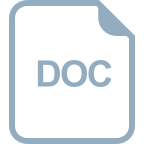
