给代码加缓存 public Result getOrderSettingByMonth(@RequestParam Integer year, @RequestParam Integer month){ System.out.println("年月:"+year+month); List<OrderSetting> orderSetting = ordersettingSrevice.showOrderSetting(year,month); List<Map<String,Object>> list = new ArrayList<>(); for (OrderSetting setting : orderSetting) { Calendar calendar = Calendar.getInstance(); calendar.setTime(setting.getOrderDate()); Map<String,Object> map = new HashMap<>(); map.put("date",calendar.get(Calendar.DAY_OF_MONTH)); map.put("number",setting.getNumber()); map.put("reservations",setting.getReservations()); list.add(map); } return new Result(true,MessageConst.GET_ORDERSETTING_SUCCESS,list); }
时间: 2023-07-16 21:17:35 浏览: 164
可以使用缓存来优化该方法,减少数据库查询次数。可以使用 Redis 或 Ehcache 等缓存框架。
以下是使用 Spring Cache 和 Ehcache 的示例代码:
首先,添加 Ehcache 依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
<dependency>
<groupId>net.sf.ehcache</groupId>
<artifactId>ehcache</artifactId>
</dependency>
```
接着,在 Spring Boot 的配置文件中添加以下配置:
```yaml
spring:
cache:
type: ehcache
cache-names: ordersettingCache
ehcache:
config: classpath:ehcache.xml
```
其中,`ordersettingCache` 是缓存的名称。
然后,创建 Ehcache 的配置文件 `ehcache.xml`:
```xml
<ehcache xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="http://www.ehcache.org/ehcache.xsd">
<cache name="ordersettingCache"
maxEntriesLocalHeap="1000"
timeToLiveSeconds="1800">
</cache>
</ehcache>
```
其中,`maxEntriesLocalHeap` 表示缓存的最大条目数,`timeToLiveSeconds` 表示缓存的过期时间(单位为秒)。
最后,在服务层方法上添加 `@Cacheable` 注解:
```java
@Service
public class OrdersettingServiceImpl implements OrdersettingService {
@Autowired
private OrdersettingMapper ordersettingMapper;
@Override
@Cacheable(value = "ordersettingCache", key = "#year + '-' + #month")
public List<OrderSetting> showOrderSetting(Integer year, Integer month) {
return ordersettingMapper.showOrderSetting(year, month);
}
}
```
其中,`@Cacheable` 注解可以指定缓存的名称和缓存的 key,这里使用了 `#year + '-' + #month` 作为缓存的 key。
这样,在调用 `getOrderSettingByMonth` 方法时,如果缓存中有对应的结果,则直接返回缓存的结果,否则执行方法体,并将结果加入缓存中。
阅读全文
相关推荐
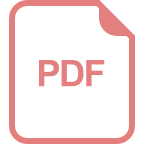
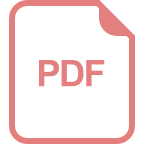
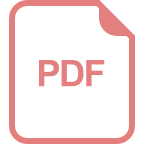

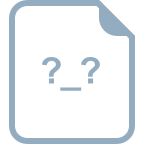
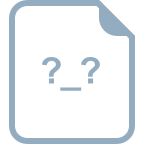
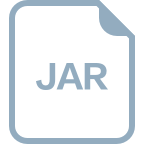
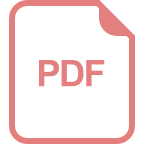
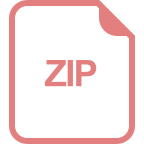
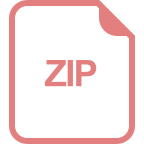
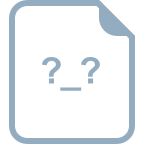
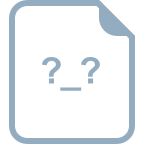
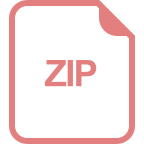
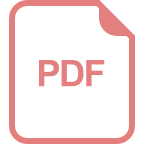
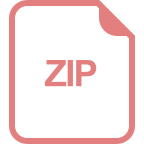
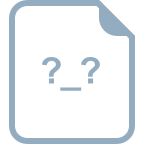
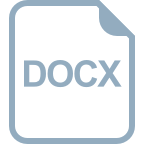
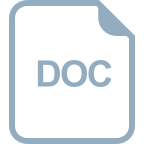
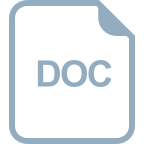
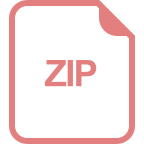