C#Datagridview判断两行是否一致
时间: 2023-07-12 16:21:34 浏览: 75
要判断两行是否一致,需要比较它们的每一列数据是否相同。你可以使用DataGridView的Cells属性获取行中的单元格数据,然后逐一比较它们的值。以下是一个示例代码:
```csharp
bool IsRowsIdentical(DataGridViewRow row1, DataGridViewRow row2)
{
if (row1.Cells.Count != row2.Cells.Count)
{
return false; // 行的单元格数不一致,不相同
}
for (int i = 0; i < row1.Cells.Count; i++)
{
if (!row1.Cells[i].Value.Equals(row2.Cells[i].Value))
{
return false; // 某个单元格的值不相同,不相同
}
}
return true; // 所有单元格的值都相同,相同
}
```
使用示例:
```csharp
// 假设dgv是一个DataGridView对象
bool isIdentical = IsRowsIdentical(dgv.Rows[0], dgv.Rows[1]);
if (isIdentical)
{
Console.WriteLine("两行数据相同");
}
else
{
Console.WriteLine("两行数据不相同");
}
```
相关问题
C#Datagridview两行数据合并
在 C# 的 DataGridView 中,可以使用 CellPainting 事件实现两行数据的合并。以下是代码示例:
```csharp
private void dataGridView1_CellPainting(object sender, DataGridViewCellPaintingEventArgs e)
{
// 判断是否需要合并
if (e.RowIndex > 0 && dataGridView1.Rows[e.RowIndex].Cells[e.ColumnIndex].Value == dataGridView1.Rows[e.RowIndex - 1].Cells[e.ColumnIndex].Value)
{
e.AdvancedBorderStyle.Top = DataGridViewAdvancedCellBorderStyle.None;
}
else
{
e.AdvancedBorderStyle.Top = dataGridView1.AdvancedCellBorderStyle.Top;
}
}
```
在上述代码中,我们判断了当前单元格是否需要与上一行合并,如果需要,则将上边框的样式设置为 None,实现两行数据的合并。
C#DataGridView判断单元格编辑时候是否按下回车
您可以使用DataGridView的EditingControlShowing事件来获取编辑控件,并向它添加一个KeyDown事件。在KeyDown事件中,您可以检查是否按下了回车键,并执行相应的操作。以下是一个示例代码:
```csharp
private void dataGridView1_EditingControlShowing(object sender, DataGridViewEditingControlShowingEventArgs e)
{
if (dataGridView1.CurrentCell.ColumnIndex == 0) // 假设只有第一列需要检查
{
TextBox textBox = e.Control as TextBox;
if (textBox != null)
{
// 添加KeyDown事件
textBox.KeyDown -= TextBox_KeyDown;
textBox.KeyDown += TextBox_KeyDown;
}
}
}
private void TextBox_KeyDown(object sender, KeyEventArgs e)
{
if (e.KeyCode == Keys.Enter)
{
// 执行您想要的操作
// ...
e.Handled = true; // 防止继续输入回车
}
}
```
请注意,此方法将检查单元格编辑控件是否为TextBox。如果使用的是其他编辑控件,您需要相应地修改代码。
相关推荐
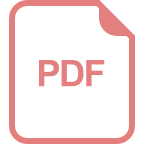
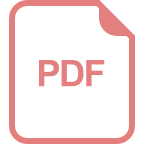












