c++用array模板定义一个二维数组
时间: 2024-05-25 14:01:36 浏览: 109
template<typename T, size_t ROWS, size_t COLS>
class Array2D {
public:
Array2D() { }
T& operator()(size_t row, size_t col) { return data[row][col]; }
const T& operator()(size_t row, size_t col) const { return data[row][col]; }
private:
T data[ROWS][COLS];
};
相关问题
二维数组形式的类模板怎么定义且输入输出
定义一个二维数组形式的类模板,可以使用如下的代码:
```c++
template<typename T, int rows, int cols>
class Array2D
{
private:
T data[rows][cols];
public:
Array2D() {}
T* operator[](int row)
{
return data[row];
}
const T* operator[](int row) const
{
return data[row];
}
void print() const
{
for (int i = 0; i < rows; ++i)
{
for (int j = 0; j < cols; ++j)
{
std::cout << data[i][j] << " ";
}
std::cout << std::endl;
}
}
};
```
该类模板使用两个非类型模板参数 `rows` 和 `cols` 分别表示二维数组的行数和列数,使用 `T` 表示数组元素的类型。该类模板中重载了 `[]` 运算符,可以用于访问二维数组中的元素。同时,还提供了一个 `print()` 成员函数,用于输出二维数组中的元素。
使用该类模板可以定义一个二维数组对象,例如:
```c++
Array2D<int, 3, 4> arr;
```
这将定义一个行数为 3,列数为 4,元素类型为 `int` 的二维数组对象 `arr`。可以使用 `[]` 运算符访问该数组中的元素,例如:
```c++
arr[0][0] = 1;
arr[0][1] = 2;
arr[1][0] = 3;
arr[1][1] = 4;
```
可以使用 `print()` 成员函数输出该数组中的元素,例如:
```c++
arr.print();
```
输出结果为:
```
1 2 0 0
3 4 0 0
0 0 0 0
```
如何用vector创建二维数组
在C++中,你可以使用`std::vector`来动态创建二维数组。这通常通过嵌套`vector`来实现,因为`vector`是一种动态数组。以下是创建二维`vector<int>`的基本步骤:
1. 首先,定义一个二维向量的模板,例如用于存储整数:
```cpp
template <typename T>
std::vector<std::vector<T>> createDynamicArray(int rows, int cols) {
```
2. 然后,在函数体内,根据传入的行数和列数初始化内部的`vector`:
```cpp
std::vector<std::vector<T>> dynamicArray(rows, std::vector<T>(cols));
```
这里,`rows`表示行数,`cols`表示列数,内外两层`vector`分别代表行和列。
3. 如果需要分配具体的值,可以遍历并设置元素:
```cpp
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
dynamicArray[i][j] = i * cols + j; // 示例,设置每个元素的初始值
}
}
4. 最后返回这个动态二维数组:
```cpp
return dynamicArray;
```
现在你可以像操作普通二维数组一样使用这个`dynamicArray`了。
阅读全文
相关推荐
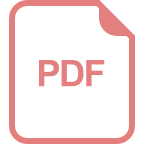
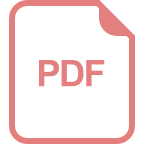
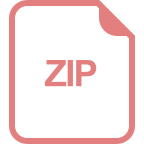
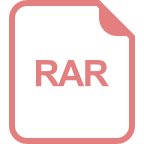
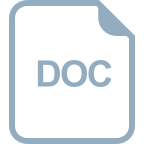
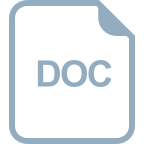
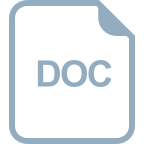
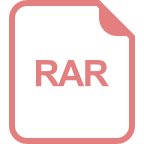
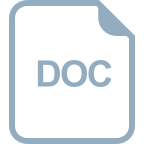
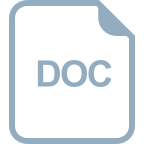
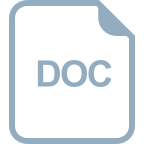
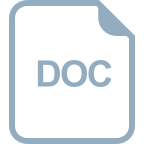
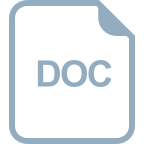
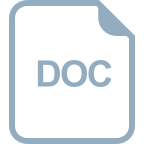
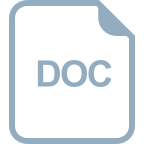
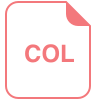