用python写一个用凹点匹配分割算法实现图像分割的代码
时间: 2024-09-08 19:04:23 浏览: 53
在Python中,你可以使用`skimage`库中的轮廓检测功能结合凹点匹配算法来实现图像分割。这里是一个简单的示例,使用了`contours`模块来找到图像中的边缘,并通过比较轮廓之间的凹点来识别潜在的区域边界:
```python
from skimage import measure, img_as_ubyte
import cv2
def find_contours(img):
# 转换为灰度图像并二值化
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
_, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
# 寻找轮廓
contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
return contours
def convex_hull_points(contour):
hull = measure.convex_hull(contour)
return contour[hull]
def find_split_points(contours):
split_points = []
for i in range(len(contours)):
contour1 = contours[i]
for j in range(i+1, len(contours)):
contour2 = contours[j]
if len(convex_hull_points(contour1)) > 3 and len(convex_hull_points(contour2)) > 3:
intersection = cv2.convexHull(cv2.findIntersection(convex_hull_points(contour1), convex_hull_points(contour2)))
if intersection is not None and len(intersection) > 2:
split_points.append(intersection)
return split_points
# 使用示例
img = cv2.imread('your_image.jpg', 0) # 替换为你想要处理的图片路径
contours = find_contours(img)
split_points = find_split_points(contours)
# 根据分割点对原图进行切割
for point_set in split_points:
x, y, w, h = cv2.boundingRect(point_set)
cropped_img = img[y:y+h, x:x+w]
# 对每个小块做进一步处理,如存储、分析等...
# 结果显示或保存
cv2.imshow('Image with Split Points', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请注意,这个代码片段是一个基本的示例,实际应用中可能需要根据图像的特点进行调整,例如噪声去除、细化分割点等。同时,如果你的图像中有复杂的形状或者多个物体需要分割,这可能不足以得到理想的效果。
阅读全文
相关推荐
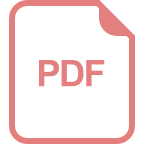
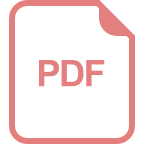


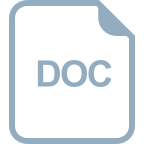
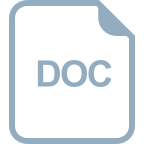




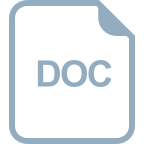
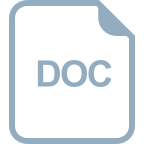
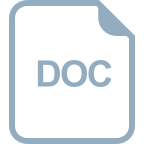
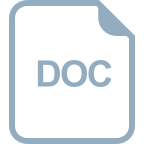
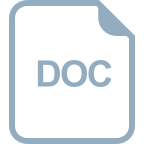


