编程实现文件合并和去重
时间: 2024-06-13 07:04:49 浏览: 16
为了实现文件合并和去重,可以使用MapReduce编程方法。具体步骤如下:
1. Map阶段:将输入文件A和B中的每一行作为一个键值对,其中键为文件中的每一行,值为一个空字符串。将这些键值对传递给Reduce阶段。
2. Reduce阶段:将Map阶段传递过来的键值对中的键作为输出的键,将值设为一个空字符串。这样,Reduce阶段的输出就是输入文件A和B中的所有不重复的行。
3. 将Reduce阶段的输出写入输出文件C中。
需要注意的是,在Map阶段和Reduce阶段都需要对输入文件进行解析,以获取每一行的内容。同时,在Reduce阶段需要进行去重操作,以确保输出文件中没有重复的行。
相关问题
MapReduce编程实现文件合并和去重操作
MapReduce是一种分布式计算模型,可以用于大规模数据处理。文件合并和去重是MapReduce中的经典案例之一。下面是基于Hadoop的MapReduce编程实现文件合并和去重操作的步骤:
1. Map阶段:将文件中的每一行作为一个输入键值对,其中键为文件名,值为文件中的一行数据。Mapper函数将键值对中的值作为输出键值对的键,将空值作为输出键值对的值,以此来完成去重操作。
2. Reduce阶段:Reducer函数将Map阶段输出的键值对中的键作为输出键值对的键,将空值作为输出键值对的值,以此来完成文件合并操作。
下面是代码实现:
```java
public class FileMergeDeduplication {
public static class Map extends Mapper<LongWritable, Text, Text, NullWritable> {
private Text outKey = new Text();
public void map(LongWritable key, Text value, Context context)
throws IOException, InterruptedException {
String line = value.toString();
outKey.set(line);
context.write(outKey, NullWritable.get());
}
}
public static class Reduce extends Reducer<Text, NullWritable, Text, NullWritable> {
public void reduce(Text key, Iterable<NullWritable> values, Context context)
throws IOException, InterruptedException {
context.write(key, NullWritable.get());
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "FileMergeDeduplication");
job.setJarByClass(FileMergeDeduplication.class);
job.setMapperClass(Map.class);
job.setReducerClass(Reduce.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(NullWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
在以上代码中,Mapper类中的`map`函数将输入的每一行作为输出键值对的键,将空值作为输出键值对的值。Reducer类中的`reduce`函数直接将输入的键值对输出即可。最后在`main`函数中设置输入路径和输出路径,并等待MapReduce任务完成。
运行以上代码,在Hadoop集群上进行MapReduce计算,即可完成文件合并和去重操作。
mapreduce编程实现文件合并和去重操作
### 回答1:
MapReduce编程可以实现文件合并和去重操作。具体步骤如下:
1. 首先,将需要合并和去重的文件上传到Hadoop分布式文件系统(HDFS)中。
2. 接着,编写MapReduce程序,其中Mapper阶段的任务是将输入文件中的每一行作为key,value为空的键值对输出。Reducer阶段的任务是将相同的key合并,并去除重复的value。
3. 在MapReduce程序中,需要设置输入路径和输出路径。输入路径为需要合并和去重的文件所在的HDFS目录,输出路径为合并和去重后的文件所在的HDFS目录。
4. 运行MapReduce程序,等待程序执行完毕。
5. 最后,从输出路径中获取合并和去重后的文件,即可完成文件合并和去重操作。
需要注意的是,在MapReduce程序中,需要设置合适的Reducer数量,以保证程序的性能和效率。同时,还需要考虑到数据倾斜等问题,采取相应的优化措施,以提高程序的运行效率。
### 回答2:
MapReduce是一种在数据处理领域广泛使用的编程模型。本文将讨论如何使用MapReduce实现文件合并和去重操作。
文件合并:
假设有多个文件需要合并成一个文件。我们可以将每个文件映射到一个键值对中,其中键表示文件名,值表示文件内容。然后通过Reduce函数将所有值合并到一个文件中。
具体实现步骤如下:
1. 将每个文件映射到一个键值对中。键为文件名,值为文件内容。
2. 将所有键值对按照键进行排序。
3. 在Reduce函数中,将所有值合并到一个文件中。
具体代码如下:
map(key, value):
# 将每个文件映射到一个键值对中
emit(key, value)
reduce(key, values):
# 将所有值合并到一个文件中
with open(key, "wb") as outfile:
for value in values:
outfile.write(value)
文件去重:
假设有多个文件中的记录存在重复数据,需要将其去重。我们可以将每个记录映射到一个键值对中,其中键表示记录的内容,值为1。然后通过Reduce函数将所有值合并到一个文件中,去除重复数据。
具体实现步骤如下:
1. 将每个记录映射到一个键值对中。键为记录的内容,值为1。
2. 在Reduce函数中,将所有值累加起来,去除值大于1的记录。
具体代码如下:
map(key, value):
# 将每个记录映射到键值对中
emit(key, 1)
reduce(key, values):
# 去除重复记录
count = 0
for value in values:
count += value
if count == 1:
emit(key, "")
### 回答3:
MapReduce编程模型是处理大规模数据集的强大工具,可以帮助我们快速地完成文件合并和去重操作。文件合并和去重操作是企业中日常工作中非常常见的操作,特别是对于需要处理海量数据的企业而言,这些操作尤为重要。
文件合并操作:
MapReduce编程模型的文件合并操作可以分为两个步骤——map和reduce。
1. Map操作:
Map操作的核心是将文件中的每一行都作为一个key-value对,将每个key相同的value按顺序组合成一组,作为reduce操作的输入。对于大规模的数据集,我们可以将数据分为多个不同的部分,每部分都运行一个独立的mapper,将处理结果输出到文件系统上。
2. Reduce操作:
Reduce操作的主要目的是将经过Map操作后产生的key-value对按照某种规则聚合在一起,用于生成最终的输出文件。对于需要进行文件合并的场景,最终输出的文件是由多个经过合并后的小文件组成。
去重操作:
去重操作与文件合并类似,也可以使用MapReduce编程模型,需要分为两个步骤——map和reduce。
1. Map操作:
Map操作的核心是将重复的数据进行分组。对于map操作,我们可以将数据集中所有的value都设为一个常数,这样检测重复值只需要比较key即可。在map操作中,如果发现有相同的key-value对,就将其过滤掉,只传递其中一个给reduce操作。
2. Reduce操作:
Reduce操作的主要功能是将来自不同mapper的处理结果聚合起来,生成最终的输出结果。在去重操作中,reduce操作会输出所有的不重复的数据。
总而言之,MapReduce编程模型可以帮助我们快速地完成文件合并和去重操作,扩大了我们处理大规模数据集的能力,也有助于加快企业的数据处理效率。
相关推荐
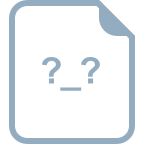
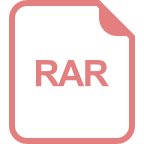
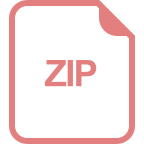












