用C++编写程序:设计一个father类、一个mother类和一个child类,其中child类继承father类和mother类。father类和mother类都包含姓和名两个数据成员,child类仅包含名数据成员。要求一个child类的对象能够输出其父、其母和自己的姓名
时间: 2024-05-06 21:18:01 浏览: 116
下面是一个简单的 C++ 程序,实现了上述要求:
```cpp
#include <iostream>
#include <string>
using namespace std;
class father {
protected:
string firstname;
string lastname;
public:
father(string fn, string ln) {
firstname = fn;
lastname = ln;
}
void printName() {
cout << "Father: " << firstname << " " << lastname << endl;
}
};
class mother {
protected:
string firstname;
string lastname;
public:
mother(string fn, string ln) {
firstname = fn;
lastname = ln;
}
void printName() {
cout << "Mother: " << firstname << " " << lastname << endl;
}
};
class child : public father, public mother {
protected:
string firstname;
public:
child(string fn, string ffn, string fln, string mfn, string mln) : father(ffn, fln), mother(mfn, mln) {
firstname = fn;
}
void printName() {
cout << "Child: " << firstname << endl;
father::printName();
mother::printName();
}
};
int main() {
child c("Alice", "Bob", "Smith", "Carol", "Johnson");
c.printName();
return 0;
}
```
在上面的程序中,我们定义了三个类:father、mother 和 child。father 和 mother 类中都包含一个 firstname 和一个 lastname 数据成员,用于存储名字和姓氏。child 类继承了 father 和 mother 类,并且只包含一个 firstname 数据成员,用于存储名字。
在 child 类的构造函数中,我们调用了 father 和 mother 类的构造函数,来初始化 firstname 和 lastname 数据成员。在 printName 函数中,我们首先输出 child 的名字,然后调用 father 和 mother 类的 printName 函数,来输出父亲和母亲的名字。
在 main 函数中,我们创建了一个 child 对象,并调用了它的 printName 函数,来输出父亲、母亲和自己的名字。运行程序,输出如下:
```
Child: Alice
Father: Bob Smith
Mother: Carol Johnson
```
阅读全文
相关推荐
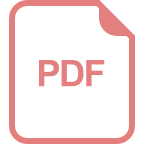






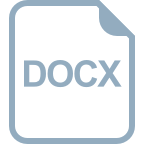
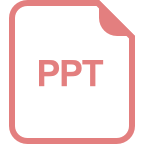
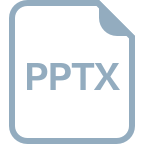
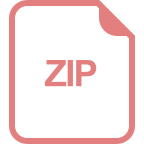
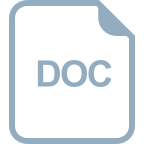
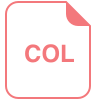




