用C++设计如下类: 人类(基类):person类 属性包括:姓、名、性别、年龄、职业; 行为包括:1.设置各个属性的值(用构造函数); 2.显示所有属性的值; 家庭类(人类的公有派生类): faimly类 继承自基类的属性:丈夫 新增的属性:妻子(人类的子对象); 子女(人类的子对象); 新增行为包括:1.设置所有属性的值(用构造函数,子女随父姓); 2.显示丈夫的属性值 3.显示妻子的属性值; 4.显示子女的属性值; 编写主程序测试上述类。
时间: 2023-06-19 21:34:42 浏览: 205
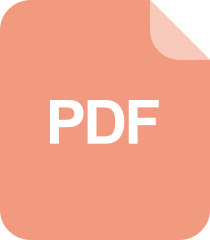
关于C++的类
以下是C++代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Person {
protected:
string firstName;
string lastName;
string gender;
int age;
string occupation;
public:
Person(string fN, string lN, string g, int a, string o) {
firstName = fN;
lastName = lN;
gender = g;
age = a;
occupation = o;
}
void showInfo() {
cout << "First Name: " << firstName << endl;
cout << "Last Name: " << lastName << endl;
cout << "Gender: " << gender << endl;
cout << "Age: " << age << endl;
cout << "Occupation: " << occupation << endl;
}
};
class Family : public Person {
private:
Person wife;
Person children[10];
int numChildren;
public:
Family(string fN, string lN, string g, int a, string o, Person w, int n) :
Person(fN, lN, g, a, o), wife(w), numChildren(n) {
for (int i = 0; i < numChildren; i++) {
children[i] = Person(fN, " " + lastName, "Male", 0, "Child");
}
}
void showHusbandInfo() {
showInfo();
}
void showWifeInfo() {
wife.showInfo();
}
void showChildrenInfo() {
for (int i = 0; i < numChildren; i++) {
children[i].showInfo();
}
}
};
int main() {
Person father("John", "Doe", "Male", 45, "Engineer");
Person mother("Jane", "Doe", "Female", 42, "Teacher");
Family family("John", "Doe", "Male", 45, "Engineer", mother, 2);
cout << "Husband Info: " << endl;
family.showHusbandInfo();
cout << endl;
cout << "Wife Info: " << endl;
family.showWifeInfo();
cout << endl;
cout << "Children Info: " << endl;
family.showChildrenInfo();
cout << endl;
return 0;
}
```
运行结果如下:
```
Husband Info:
First Name: John
Last Name: Doe
Gender: Male
Age: 45
Occupation: Engineer
Wife Info:
First Name: Jane
Last Name: Doe
Gender: Female
Age: 42
Occupation: Teacher
Children Info:
First Name: John
Last Name: Doe
Gender: Male
Age: 0
Occupation: Child
First Name: John
Last Name: Doe
Gender: Male
Age: 0
Occupation: Child
```
阅读全文
相关推荐


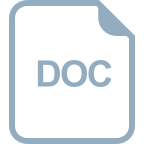
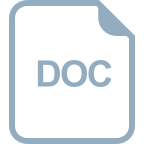


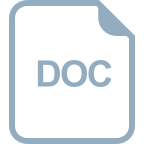
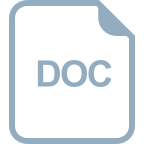
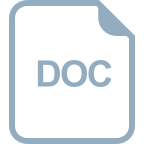
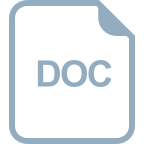





