用c++实现:编写程序测试如下类: 人类(基类): 属性包括姓名、性别、年龄、职业; 行为包括:1.设置各个属性值(用构造函数); 2.显示某个属性值; 3.显示所有属性值; 学生类(人类的派生类):新属性有:学校、专业、年级 新的行为:1.显示某个新增的属性; 2.显示所有属性;
时间: 2023-07-15 09:13:37 浏览: 86
以下是用C++实现的代码:
```c++
#include <iostream>
#include <string>
using namespace std;
class Person {
public:
Person(string n, string s, int a, string j) : name(n), sex(s), age(a), job(j) {}
void setName(string n) { name = n; }
void setSex(string s) { sex = s; }
void setAge(int a) { age = a; }
void setJob(string j) { job = j; }
string getName() const { return name; }
string getSex() const { return sex; }
int getAge() const { return age; }
string getJob() const { return job; }
void showName() const { cout << "Name: " << name << endl; }
void showSex() const { cout << "Sex: " << sex << endl; }
void showAge() const { cout << "Age: " << age << endl; }
void showJob() const { cout << "Job: " << job << endl; }
void showAll() const {
cout << "Name: " << name << endl;
cout << "Sex: " << sex << endl;
cout << "Age: " << age << endl;
cout << "Job: " << job << endl;
}
private:
string name;
string sex;
int age;
string job;
};
class Student : public Person {
public:
Student(string n, string s, int a, string j, string sc, string m, int g) : Person(n, s, a, j), school(sc), major(m), grade(g) {}
void setSchool(string sc) { school = sc; }
void setMajor(string m) { major = m; }
void setGrade(int g) { grade = g; }
string getSchool() const { return school; }
string getMajor() const { return major; }
int getGrade() const { return grade; }
void showSchool() const { cout << "School: " << school << endl; }
void showMajor() const { cout << "Major: " << major << endl; }
void showGrade() const { cout << "Grade: " << grade << endl; }
void showAll() const {
Person::showAll();
cout << "School: " << school << endl;
cout << "Major: " << major << endl;
cout << "Grade: " << grade << endl;
}
private:
string school;
string major;
int grade;
};
int main() {
Person p("Tom", "Male", 25, "Engineer");
cout << "Person:" << endl;
p.showAll();
cout << endl;
Student s("Lucy", "Female", 20, "Student", "Zhejiang University", "Computer Science", 2);
cout << "Student:" << endl;
s.showAll();
cout << endl;
return 0;
}
```
在上面的代码中,我们首先定义了一个人类(`Person`)作为基类,包含了姓名、性别、年龄、职业等属性,以及设置和显示这些属性的方法。然后,我们定义了一个学生类(`Student`),它是人类的派生类,新增了学校、专业、年级等属性,以及显示这些属性的方法。
在主函数中,我们创建了一个人类对象和一个学生类对象,并测试了它们的行为。可以看到,在学生类中,我们通过调用基类的显示所有属性的方法来显示所有属性,这是因为学生类继承了人类的所有属性和方法,可以直接访问基类的成员。
阅读全文
相关推荐
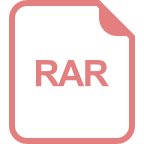
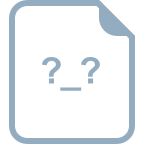
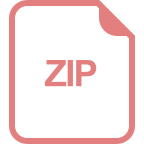
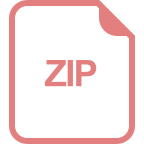
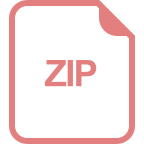
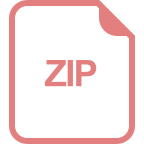
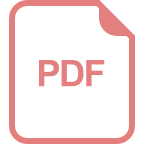
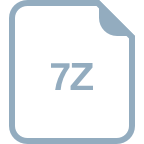
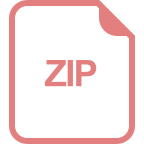
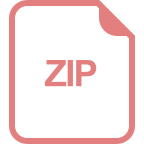
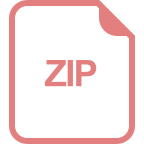
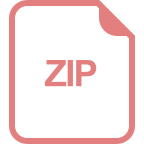
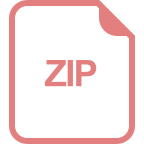
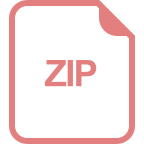
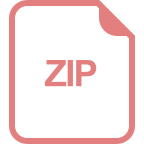
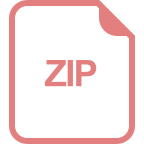