在Java后台Servlet中使用JSONObject要哪些条件,用代码说明
时间: 2024-03-03 08:50:41 浏览: 22
在Java后台Servlet中使用JSONObject,需要满足以下条件,示例代码如下:
1. 引入JSON库
使用JSONObject需要引入JSON库,如fastjson、Jackson等。
```java
import com.alibaba.fastjson.JSONObject;
```
2. 请求头中的Content-Type为application/json
使用JSONObject解析请求体中的JSON数据,需要保证请求头中的Content-Type为application/json,否则无法正确解析请求体数据。
```java
String contentType = request.getHeader("Content-Type");
if (contentType == null || !contentType.contains("application/json")) {
// Content-Type不是application/json,无法解析请求体数据
return;
}
```
3. 请求体中为合法的JSON格式数据
使用JSONObject解析请求体数据,需要保证请求体中为合法的JSON格式数据,否则无法正确解析。
```java
BufferedReader reader = request.getReader();
String line = null;
StringBuilder sb = new StringBuilder();
while ((line = reader.readLine()) != null) {
sb.append(line);
}
String requestBody = sb.toString();
try {
JSONObject jsonObject = JSONObject.parseObject(requestBody);
} catch (JSONException e) {
// 请求体中不是合法的JSON格式数据
return;
}
```
4. 请求体中的JSON数据项名称和Java对象属性名称一致
使用JSONObject将请求体数据解析成Java对象时,需要确保请求体中的JSON数据项名称和Java对象属性名称一致,否则无法正确解析。
```java
class RequestData {
private String dataItem;
public String getDataItem() {
return dataItem;
}
public void setDataItem(String dataItem) {
this.dataItem = dataItem;
}
}
BufferedReader reader = request.getReader();
String line = null;
StringBuilder sb = new StringBuilder();
while ((line = reader.readLine()) != null) {
sb.append(line);
}
String requestBody = sb.toString();
try {
JSONObject jsonObject = JSONObject.parseObject(requestBody);
RequestData requestData = jsonObject.toJavaObject(RequestData.class);
String dataItem = requestData.getDataItem();
} catch (JSONException e) {
// 请求体中不是合法的JSON格式数据
return;
} catch (NullPointerException e) {
// JSONObject对象为空
return;
} catch (Exception e) {
// 其他异常
return;
}
```
5. JSONObject对象非空
使用JSONObject获取请求体中的JSON数据项时,需要确保JSONObject对象非空,否则无法获取其中的数据项。
```java
BufferedReader reader = request.getReader();
String line = null;
StringBuilder sb = new StringBuilder();
while ((line = reader.readLine()) != null) {
sb.append(line);
}
String requestBody = sb.toString();
try {
JSONObject jsonObject = JSONObject.parseObject(requestBody);
if (jsonObject != null) {
String dataItem = jsonObject.getString("dataItem");
// 处理数据项
}
} catch (JSONException e) {
// 请求体中不是合法的JSON格式数据
return;
}
```
需要注意的是,使用JSONObject时需要避免空指针异常和其他异常,建议在使用之前进行相关的判断和异常处理。
相关推荐
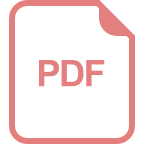
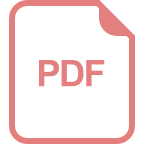














