请使用C语言编写一个程序,在linux环境下实现多进程文件复制功能,要求用户从命令行输入源文件名和目标文件名字,并使用多个子进程将源文件内容复制到目文件中,每个子进程负责源文件的一部分,子进程之间通过管道惊醒通信,协调复制工作
时间: 2024-03-05 19:50:05 浏览: 69
好的,下面是实现多进程文件复制功能的C语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <fcntl.h>
#define BUFSIZE 1024
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("Usage: %s <source file> <target file>\n", argv[0]);
exit(EXIT_FAILURE);
}
char *src_file = argv[1];
char *tgt_file = argv[2];
// 打开源文件
int src_fd = open(src_file, O_RDONLY);
if (src_fd < 0) {
perror("open source file error");
exit(EXIT_FAILURE);
}
// 创建目标文件
int tgt_fd = open(tgt_file, O_CREAT | O_WRONLY, 0666);
if (tgt_fd < 0) {
perror("create target file error");
exit(EXIT_FAILURE);
}
// 获取源文件大小
off_t file_size = lseek(src_fd, 0, SEEK_END);
if (file_size < 0) {
perror("get source file size error");
exit(EXIT_FAILURE);
}
// 计算每个子进程需要复制的字节数
int chunk_size = file_size / 4;
// 创建管道
int pipefd[2];
if (pipe(pipefd) != 0) {
perror("create pipe error");
exit(EXIT_FAILURE);
}
// 创建子进程
for (int i = 0; i < 4; i++) {
pid_t pid = fork();
if (pid < 0) {
perror("create child process error");
exit(EXIT_FAILURE);
} else if (pid == 0) {
// 子进程
close(pipefd[0]); // 关闭读端
// 每个子进程负责复制源文件的一部分
off_t start = i * chunk_size;
off_t end = (i == 3) ? file_size : ((i + 1) * chunk_size);
char buf[BUFSIZE];
int read_bytes, write_bytes;
// 移动文件读写指针到起始位置
lseek(src_fd, start, SEEK_SET);
lseek(tgt_fd, start, SEEK_SET);
// 从源文件读取内容,并写入目标文件
while ((read_bytes = read(src_fd, buf, BUFSIZE)) > 0) {
if (start + read_bytes > end) {
// 如果读取的字节数超过子进程负责的范围,则截取部分内容
read_bytes = end - start;
}
write_bytes = write(tgt_fd, buf, read_bytes);
if (write_bytes != read_bytes) {
perror("write target file error");
exit(EXIT_FAILURE);
}
start += read_bytes;
if (start >= end) {
break;
}
}
close(pipefd[1]); // 关闭写端
exit(EXIT_SUCCESS);
}
}
close(pipefd[1]); // 关闭写端
// 等待子进程结束
for (int i = 0; i < 4; i++) {
wait(NULL);
}
close(src_fd);
close(tgt_fd);
printf("File copy completed!\n");
return 0;
}
```
程序主要实现了以下几个步骤:
1. 从命令行参数中获取源文件名和目标文件名;
2. 打开源文件和创建目标文件;
3. 获取源文件大小,计算每个子进程需要复制的字节数;
4. 创建管道和四个子进程,每个子进程负责复制源文件的一部分;
5. 等待子进程结束并关闭文件描述符;
6. 打印复制完成的提示信息。
注意,程序中使用了 `fork()` 函数创建子进程,使用了 `pipe()` 函数创建管道,使用了 `wait()` 函数等待子进程结束,使用了 `lseek()` 函数移动文件读写指针,使用了 `read()` 和 `write()` 函数读写文件内容。
阅读全文
相关推荐


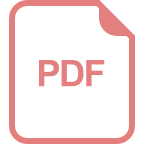
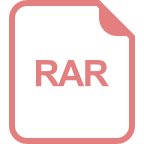
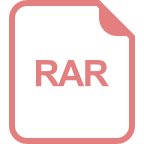
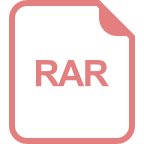


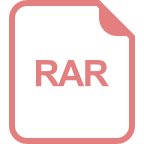
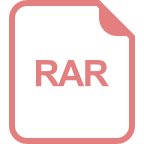
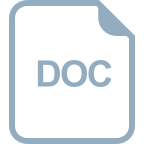





