编写一个程序,实现文件复制。(源文件和目标文件名从键盘输入)。要求用c++实现
时间: 2023-08-31 13:20:46 浏览: 55
### 回答1:
以下是一个简单的 C++ 程序,可以实现文件复制功能,其中源文件和目标文件名从键盘输入:
```cpp
#include <iostream>
#include <fstream>
using namespace std;
int main() {
string source_file, target_file;
cout << "请输入源文件名:";
cin >> source_file;
cout << "请输入目标文件名:";
cin >> target_file;
ifstream source(source_file, ios::binary);
if (!source) {
cout << "打开源文件失败!" << endl;
return 1;
}
ofstream target(target_file, ios::binary);
if (!target) {
cout << "创建目标文件失败!" << endl;
return 1;
}
char buffer[1024];
while (source.read(buffer, sizeof(buffer))) {
target.write(buffer, sizeof(buffer));
}
target.write(buffer, source.gcount());
source.close();
target.close();
cout << "文件复制完成!" << endl;
return 0;
}
```
程序中使用了 `ifstream` 和 `ofstream` 类来分别读取源文件和写入目标文件,其中 `ios::binary` 参数表示以二进制方式读写文件。程序中使用了一个 1024 字节的缓冲区,每次从源文件中读取 1024 字节数据,并写入目标文件,直到源文件读取完毕。最后,程序关闭源文件和目标文件,并输出完成提示信息。
### 回答2:
编写一个程序实现文件复制可以按以下步骤进行:
1. 首先,使用#include <stdio.h>预处理指令导入标准输入输出库。
2. 在主函数中定义源文件名和目标文件名的字符数组变量。
3. 通过使用printf函数提示用户输入源文件名和目标文件名,并使用scanf函数接收用户输入的文件名。
4. 使用FILE类型的指针变量来打开源文件和目标文件。源文件使用只读模式打开(“r”),目标文件使用写入模式打开(“w”)。
5. 判断源文件和目标文件是否成功打开。若打开失败,则使用perror函数输出错误信息并终止程序。
6. 使用fgetc函数从源文件中读取字符,并使用fputc函数将字符写入目标文件,直到源文件的末尾(使用EOF检查)。
7. 关闭源文件和目标文件。关闭文件可以使用fclose函数。
下面是一个示例代码:
```c
#include <stdio.h>
int main() {
char sourceFileName[100];
char targetFileName[100];
FILE *sourceFile;
FILE *targetFile;
char ch;
printf("请输入源文件名:");
scanf("%s", sourceFileName);
printf("请输入目标文件名:");
scanf("%s", targetFileName);
sourceFile = fopen(sourceFileName, "r");
targetFile = fopen(targetFileName, "w");
if (sourceFile == NULL || targetFile == NULL) {
perror("文件打开失败");
return -1;
}
while ((ch = fgetc(sourceFile)) != EOF) {
fputc(ch, targetFile);
}
fclose(sourceFile);
fclose(targetFile);
printf("文件复制成功\n");
return 0;
}
```
在这个示例代码中,首先提示用户输入源文件名和目标文件名,然后打开这两个文件。通过使用循环从源文件中读取字符并将其写入目标文件,实现文件复制。最后关闭源文件和目标文件,并输出复制成功的提示信息。
### 回答3:
下面是一个使用C语言编写的实现文件复制的程序:
```c
#include <stdio.h>
int main() {
char sourceFile[50], targetFile[50];
FILE *source, *target;
char ch;
printf("请输入源文件名:");
scanf("%s", sourceFile);
printf("请输入目标文件名:");
scanf("%s", targetFile);
source = fopen(sourceFile, "r");
if (source == NULL) {
printf("无法打开源文件\n");
return 1;
}
target = fopen(targetFile, "w");
if (target == NULL) {
printf("无法打开目标文件\n");
fclose(source);
return 1;
}
while ((ch = fgetc(source)) != EOF) {
fputc(ch, target);
}
printf("文件复制成功\n");
fclose(source);
fclose(target);
return 0;
}
```
以上程序运行后,会要求用户输入源文件名和目标文件名,然后打开源文件并读取其中的内容,将内容逐字节地写入目标文件中,从而实现文件的复制。如果无法打开源文件或目标文件,则会输出相应错误信息。最后,输出文件复制成功的提示,并关闭源文件和目标文件。
请注意,文件的路径需要与该程序的路径相符,否则需要提供文件的完整路径才能成功打开。
相关推荐
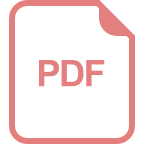
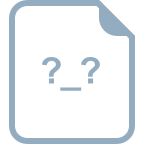














