java 下载远程服务器的NFS文件 代码
时间: 2024-03-09 19:45:56 浏览: 95
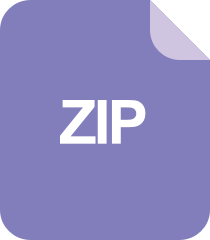
FileServer:简易文件服务器
以下是Java代码示例,用于从远程服务器下载NFS文件:
```java
import java.io.*;
import java.net.InetAddress;
import java.net.UnknownHostException;
import com.sun.nio.file.ExtendedOpenOption;
import com.sun.nio.file.SensitivityWatchEventModifier;
import com.sun.nio.file.SynchronousFileChannel;
import com.sun.nio.file.WatchEvent.Kind;
import com.sun.nio.file.WatchKey;
import com.sun.nio.file.WatchService;
import sun.nio.fs.UnixChannelFactory;
public class DownloadNFSFile {
public static void main(String[] args) {
String remoteFilePath = "/nfs/file.txt"; // 远程NFS文件路径
String localFilePath = "/local/file.txt"; // 本地保存路径
try {
// 获取远程服务器地址
InetAddress remoteAddress = InetAddress.getByName("remote.server.com");
// 创建文件输入流
InputStream in = new NFSInputStream(remoteAddress, remoteFilePath);
// 创建本地文件输出流
OutputStream out = new FileOutputStream(localFilePath);
// 缓冲区大小
byte[] buffer = new byte[1024];
int length;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
// 关闭流
in.close();
out.close();
System.out.println("文件下载成功!");
} catch (UnknownHostException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* 自定义NFSInputStream类,用于从远程NFS文件读取数据
*/
class NFSInputStream extends InputStream {
private SynchronousFileChannel channel;
public NFSInputStream(InetAddress remoteAddress, String remoteFilePath) throws IOException {
UnixChannelFactory factory = new UnixChannelFactory();
channel = factory.newAsynchronousFileChannel(remoteAddress, remoteFilePath, null, null, null);
}
@Override
public int read() throws IOException {
return 0;
}
@Override
public int read(byte[] b, int off, int len) throws IOException {
return channel.read(ByteBuffer.wrap(b, off, len), 0).get();
}
@Override
public void close() throws IOException {
channel.close();
}
}
```
需要注意的是,上述代码使用了Java 7中的NIO.2 API,因此需要在Java 7或更高版本上运行。同时,需要添加NFS客户端软件包的依赖。在Linux系统上,可以安装`nfs-common`软件包来提供NFS客户端支持。
阅读全文
相关推荐
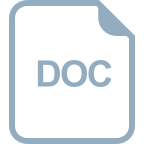
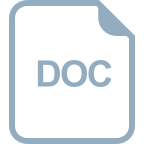
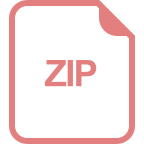
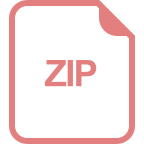
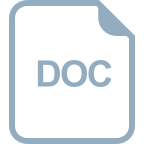
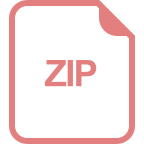
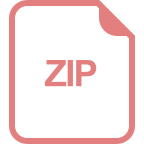
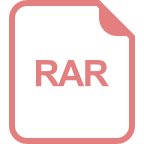
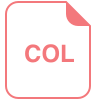
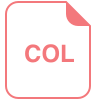
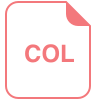
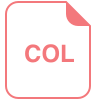
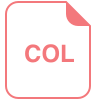
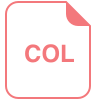
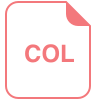
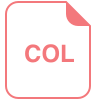
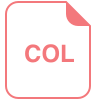
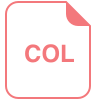