#include <bits/stdc++.h> using namespace std; class Student { protected: string name_; bool sex_; int grade_; Student next; public: Student(string name, bool sex, int grade):name(name), sex(sex),grade(grade) { cout<<"A student named by "<<name<<" is created!"<<endl; } ~Student() { cout<<"A student named by "<<name<<" is erased."<<endl; } void showInfo() { cout<<"name = "<<name<<", sex = " <<sex_<<", grade = "<<grade_<<";"; } Student getNext() { return next; } void setNext(Student* s) { next=s; } }; class StudentCadre:public Student { protected: string position_; public: StudentCadre(string name, bool sex, int grade, string pos) :Student(name,sex,grade),position(pos) { cout<<"A student cadre with position "<<position<<" is created."<<endl; } ~StudentCadre(){cout<<"A student named by "<<name<<" is erased."<<endl;} void showInfo() { cout<<"name = "<<name<<", sex = " <<sex<<", grade = "<<grade<<"; position = "<<position_<<"."; } }; int main() { int num; string name, position; bool sex; int grade; Student *header, *student, curStudent; cin>>name>>sex>>grade>>position; header = new StudentCadre(name, sex, grade,position); curStudent = header; cin>>num; for (int i = 0; i < num; i++) { cin>>name>>sex>>grade; student = new Student(name, sex, grade); curStudent -> setNext(student); curStudent = curStudent -> getNext(); } ((StudentCadre)header) -> showInfo(); cout<<endl; curStudent = header; while (curStudent -> getNext() != NULL) { curStudent = curStudent -> getNext(); curStudent->showInfo(); cout<<endl; } curStudent = header; while (curStudent != NULL) { student = curStudent; curStudent = curStudent -> getNext(); delete student; } return 0; }这段代码有什么问题
时间: 2024-01-16 09:02:21 浏览: 117
这段代码存在以下问题:
1. 在 `Student` 类中,`next` 成员变量应该是一个指向 `Student` 类型的指针,而不是 `Student` 类型本身。正确的定义应该是 `Student *next;`。
2. 在 `StudentCadre` 类中,构造函数中应该调用父类 `Student` 的构造函数,而不是尝试重新定义 `name`、`sex` 和 `grade`。正确的调用方式应该是 `Student(name, sex, grade)`。
3. 在 `StudentCadre` 类中,构造函数中应该将 `pos` 赋值给成员变量 `position_`,而不是 `position`。正确的赋值语句应该是 `position_ = pos;`。
4. 在 `main` 函数中,声明 `curStudent` 时应该是 `Student *curStudent;`,而不是 `Student curStudent;`。同时,由于 `header` 是一个指向 `Student` 类型的指针,因此在调用 `showInfo()` 函数时需要进行类型转换,即 `(StudentCadre *)header->showInfo();`。
5. 在 `main` 函数中,最后删除链表时,应该先将 `header` 的下一个节点保存下来,再删除 `header`,然后将下一个节点作为新的 `header`,直到链表为空。正确的删除链表的代码应该是:
```cpp
curStudent = header;
while (curStudent != NULL) {
student = curStudent;
curStudent = curStudent->getNext();
delete student;
}
```
以上是我发现的问题,希望对你有帮助。
阅读全文
相关推荐
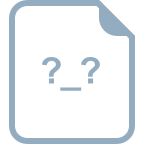
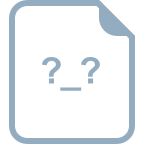
















