我需要一个使用harris算法实现两幅图片完成全景图像拼接的代码
时间: 2023-12-14 12:36:29 浏览: 34
好的,以下是使用Harris算法实现全景图像拼接的Python代码:
```python
import cv2
import numpy as np
# 读取两幅图片
img1 = cv2.imread('img1.jpg')
img2 = cv2.imread('img2.jpg')
# 转换为灰度图像
gray1 = cv2.cvtColor(img1, cv2.COLOR_BGR2GRAY)
gray2 = cv2.cvtColor(img2, cv2.COLOR_BGR2GRAY)
# 使用Harris角点检测
corners1 = cv2.cornerHarris(gray1, 2, 3, 0.04)
corners2 = cv2.cornerHarris(gray2, 2, 3, 0.04)
# 取最大值作为角点检测结果
corners1 = cv2.dilate(corners1, None)
corners2 = cv2.dilate(corners2, None)
threshold = 0.01 * max(corners1.max(), corners2.max())
corners1[corners1 < threshold] = 0
corners2[corners2 < threshold] = 0
# 寻找对应特征点
kp1 = [cv2.KeyPoint(x, y, 1) for y in range(corners1.shape[0]) for x in range(corners1.shape[1]) if corners1[y, x] != 0]
kp2 = [cv2.KeyPoint(x, y, 1) for y in range(corners2.shape[0]) for x in range(corners2.shape[1]) if corners2[y, x] != 0]
# 提取特征描述符
orb = cv2.ORB_create()
des1 = orb.compute(gray1, kp1)[1]
des2 = orb.compute(gray2, kp2)[1]
# 匹配特征点
bf = cv2.BFMatcher(cv2.NORM_HAMMING, crossCheck=True)
matches = bf.match(des1, des2)
matches = sorted(matches, key=lambda x: x.distance)
# 选取最佳匹配点
src_pts = np.float32([kp1[m.queryIdx].pt for m in matches]).reshape(-1, 1, 2)
dst_pts = np.float32([kp2[m.trainIdx].pt for m in matches]).reshape(-1, 1, 2)
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
# 拼接图像
result = cv2.warpPerspective(img1, M, (img1.shape[1] + img2.shape[1], img1.shape[0]))
result[0:img2.shape[0], 0:img2.shape[1]] = img2
cv2.imwrite('result.jpg', result)
```
注意:上述代码仅供参考,实际应用中可能需要进行一些调整和优化。
相关推荐
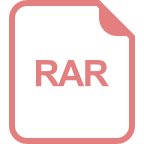
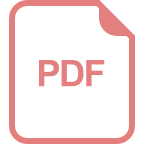
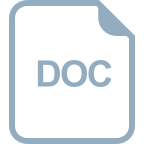







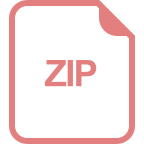
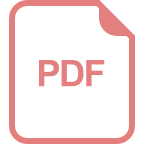
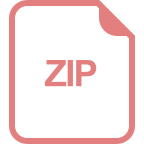
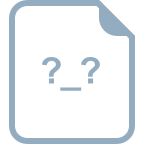
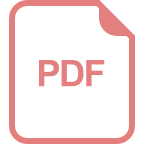
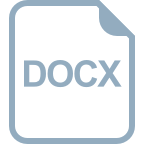
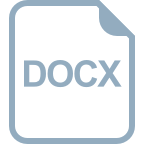