请用Java 写一个记事本,要求“文件”“编辑”“格式”“查看”“帮助”
时间: 2023-09-12 14:07:40 浏览: 109
以下是一个简单的Java记事本程序,实现了菜单栏的“文件”“编辑”“格式”“查看”“帮助”:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class JavaNotepad extends JFrame implements ActionListener {
JTextArea textArea;
JScrollPane scrollPane;
public JavaNotepad() {
setTitle("Java Notepad");
setSize(800, 600);
setLocationRelativeTo(null);
setDefaultCloseOperation(EXIT_ON_CLOSE);
JMenuBar menuBar = new JMenuBar();
JMenu fileMenu = new JMenu("文件");
JMenuItem newMenuItem = new JMenuItem("新建");
newMenuItem.addActionListener(this);
fileMenu.add(newMenuItem);
JMenuItem openMenuItem = new JMenuItem("打开");
openMenuItem.addActionListener(this);
fileMenu.add(openMenuItem);
JMenuItem saveMenuItem = new JMenuItem("保存");
saveMenuItem.addActionListener(this);
fileMenu.add(saveMenuItem);
JMenuItem exitMenuItem = new JMenuItem("退出");
exitMenuItem.addActionListener(this);
fileMenu.add(exitMenuItem);
menuBar.add(fileMenu);
JMenu editMenu = new JMenu("编辑");
JMenuItem cutMenuItem = new JMenuItem("剪切");
cutMenuItem.addActionListener(this);
editMenu.add(cutMenuItem);
JMenuItem copyMenuItem = new JMenuItem("复制");
copyMenuItem.addActionListener(this);
editMenu.add(copyMenuItem);
JMenuItem pasteMenuItem = new JMenuItem("粘贴");
pasteMenuItem.addActionListener(this);
editMenu.add(pasteMenuItem);
menuBar.add(editMenu);
JMenu formatMenu = new JMenu("格式");
JMenuItem fontMenuItem = new JMenuItem("字体");
fontMenuItem.addActionListener(this);
formatMenu.add(fontMenuItem);
menuBar.add(formatMenu);
JMenu viewMenu = new JMenu("查看");
JMenuItem zoomInMenuItem = new JMenuItem("放大");
zoomInMenuItem.addActionListener(this);
viewMenu.add(zoomInMenuItem);
JMenuItem zoomOutMenuItem = new JMenuItem("缩小");
zoomOutMenuItem.addActionListener(this);
viewMenu.add(zoomOutMenuItem);
menuBar.add(viewMenu);
JMenu helpMenu = new JMenu("帮助");
JMenuItem aboutMenuItem = new JMenuItem("关于");
aboutMenuItem.addActionListener(this);
helpMenu.add(aboutMenuItem);
menuBar.add(helpMenu);
setJMenuBar(menuBar);
textArea = new JTextArea();
scrollPane = new JScrollPane(textArea);
add(scrollPane);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
String command = e.getActionCommand();
switch (command) {
case "新建":
textArea.setText("");
break;
case "打开":
JFileChooser openFileChooser = new JFileChooser();
if (openFileChooser.showOpenDialog(this) == JFileChooser.APPROVE_OPTION) {
// 读取文件内容
}
break;
case "保存":
JFileChooser saveFileChooser = new JFileChooser();
if (saveFileChooser.showSaveDialog(this) == JFileChooser.APPROVE_OPTION) {
// 写入文件内容
}
break;
case "退出":
System.exit(0);
break;
case "剪切":
textArea.cut();
break;
case "复制":
textArea.copy();
break;
case "粘贴":
textArea.paste();
break;
case "字体":
FontChooser fontChooser = new FontChooser(this);
fontChooser.setVisible(true);
break;
case "放大":
Font currentFont = textArea.getFont();
float currentSize = currentFont.getSize2D();
textArea.setFont(currentFont.deriveFont(currentSize + 2));
break;
case "缩小":
Font currentFont2 = textArea.getFont();
float currentSize2 = currentFont2.getSize2D();
textArea.setFont(currentFont2.deriveFont(currentSize2 - 2));
break;
case "关于":
JOptionPane.showMessageDialog(this, "Java Notepad v1.0");
break;
}
}
public static void main(String[] args) {
new JavaNotepad();
}
class FontChooser extends JDialog implements ActionListener {
JComboBox<String> fontComboBox;
JComboBox<Integer> sizeComboBox;
JCheckBox boldCheckBox;
JCheckBox italicCheckBox;
JButton okButton;
JButton cancelButton;
public FontChooser(Frame owner) {
super(owner, "字体选择", true);
setSize(400, 200);
setLocationRelativeTo(null);
JPanel panel = new JPanel();
panel.setLayout(new GridLayout(4, 2));
add(panel);
fontComboBox = new JComboBox<>(GraphicsEnvironment.getLocalGraphicsEnvironment().getAvailableFontFamilyNames());
panel.add(new JLabel("字体:"));
panel.add(fontComboBox);
sizeComboBox = new JComboBox<>(new Integer[]{8, 9, 10, 11, 12, 14, 16, 18, 20, 22, 24, 26, 28, 36, 48, 72});
panel.add(new JLabel("大小:"));
panel.add(sizeComboBox);
boldCheckBox = new JCheckBox("粗体");
panel.add(boldCheckBox);
italicCheckBox = new JCheckBox("斜体");
panel.add(italicCheckBox);
okButton = new JButton("确定");
okButton.addActionListener(this);
panel.add(okButton);
cancelButton = new JButton("取消");
cancelButton.addActionListener(this);
panel.add(cancelButton);
Font currentFont = textArea.getFont();
fontComboBox.setSelectedItem(currentFont.getFamily());
sizeComboBox.setSelectedItem((int) currentFont.getSize2D());
boldCheckBox.setSelected(currentFont.isBold());
italicCheckBox.setSelected(currentFont.isItalic());
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == okButton) {
String fontName = (String) fontComboBox.getSelectedItem();
int fontSize = (int) sizeComboBox.getSelectedItem();
int fontStyle = 0;
if (boldCheckBox.isSelected()) {
fontStyle += Font.BOLD;
}
if (italicCheckBox.isSelected()) {
fontStyle += Font.ITALIC;
}
textArea.setFont(new Font(fontName, fontStyle, fontSize));
}
setVisible(false);
}
}
}
```
注意:这只是一个简单的示例程序,对于实际的记事本程序,还有很多功能需要进一步完善和实现。
阅读全文
相关推荐





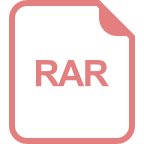
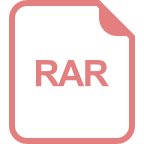
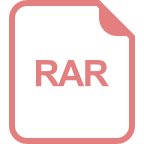


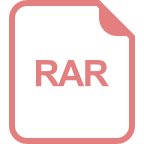
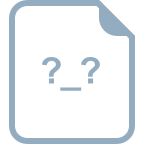






