A星算法动态路径规划,提供相关c++代码
时间: 2024-10-09 22:11:10 浏览: 43
A*算法是一种启发式搜索算法,常用于求解动态路径规划问题,如游戏中的角色寻路、机器人导航等。它结合了宽度优先搜索(广度优先搜索)的高效性和迪杰斯特拉算法(Dijkstra)的优化特性,通过估算从起点到目标点的最短距离(称为“启发函数”),优先探索更接近目标的节点。
以下是简单的C++代码实现A*算法的基本框架:
```cpp
#include <vector>
#include <queue>
#include <utility>
struct Node {
int x, y; // 节点位置
int cost; // 到源点的距离
int heuristic; // 启发函数值
bool visited = false;
Node* parent = nullptr; // 父节点指针
Node(int x, int y) : x(x), y(y), cost(0), heuristic(calculateHeuristic()) {}
};
int calculateHeuristic(Node& start, Node& end) { // 假设曼哈顿距离作为启发函数
return abs(start.x - end.x) + abs(start.y - end.y);
}
std::vector<Node*> aStarSearch(Node* start, Node* goal) {
std::priority_queue<std::pair<int, Node*>, std::vector<std::pair<int, Node*>>, std::greater<int>> pq;
pq.push({start->cost + start->heuristic, start});
while (!pq.empty()) {
auto [currentCost, currentNode] = pq.top();
pq.pop();
if (currentNode == goal) {
return constructPath(currentNode);
}
currentNode->visited = true;
for (auto neighbor : getNeighbors(currentNode)) { // 获取邻居节点
int newCost = currentCost + neighbor->cost;
if (!neighbor->visited || newCost < neighbor->cost) {
neighbor->cost = newCost;
neighbor->parent = currentNode;
neighbor->heuristic = calculateHeuristic(*neighbor, *goal);
pq.push({neighbor->cost + neighbor->heuristic, neighbor});
}
}
}
return {}; // 如果没有找到路径,返回空
}
std::vector<Node*> constructPath(Node* node) {
std::vector<Node*> path;
while (node) {
path.push_back(node);
node = node->parent;
}
reverse(path.begin(), path.end());
return path;
}
```
在这个例子中,`getNeighbors`是一个辅助函数,用于获取给定节点的所有相邻节点。注意实际应用中需要替换为具体环境下的邻接关系判断。
阅读全文
相关推荐
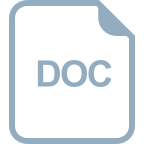
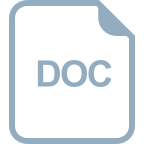
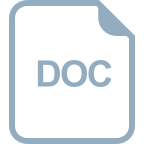
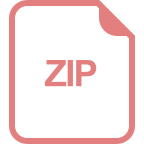
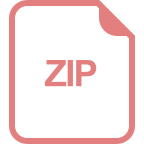
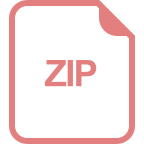
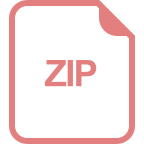
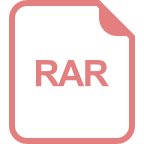
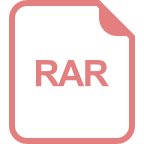
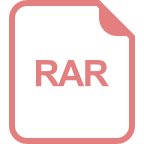
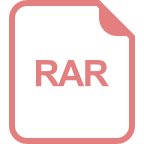
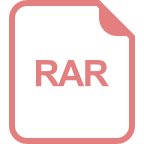
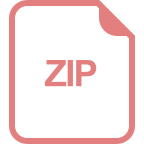
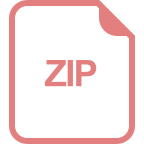
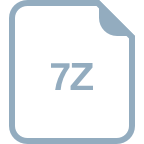
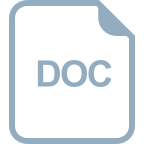
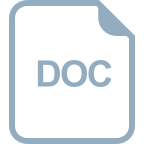
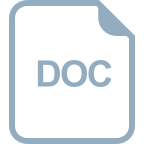
