#include <stdio.h> #include <stdlib.h> #include <string.h> #define MAX_SIZE 100
时间: 2024-06-18 08:01:30 浏览: 249
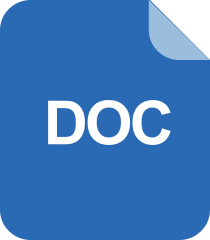
#include<stdio.h>
这个代码片段包含了三个常用的C语言库头文件:
1. **stdio.h**:标准输入输出库,提供了基本的文件操作和输入/输出功能,如printf(), scanf(), fgets()等。
2. **stdlib.h**:标准库头,包含一些数学函数、内存管理和错误处理等,比如malloc(), free(), atoi()等。
3. **string.h**:字符串处理库,提供了字符串操作相关的函数,例如strlen(), strcpy(), strcmp(), strcat()等。
`#define MAX_SIZE 100`是一个预处理器宏定义,它将字符串"MAX_SIZE"替换为常量100。这个定义通常用于设置数组或其他动态分配数据结构的最大大小,这里是100字节。在程序中,你可以使用`MAX_SIZE`来限制字符串或字符数组的最大长度,例如创建一个最多容纳100个字符的数组。
阅读全文
相关推荐
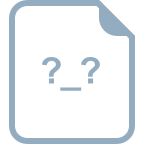
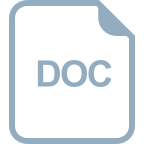
















