输入平面中的两个点A、B的坐标,(x1,y1)和(x2,y2),要求分别输出两点的坐标,最后在屏幕中显示出两点的距离。 两个点A、B坐标为(x1,y1)和(x2,y2),如图3-3所示,根据数学知识可知,两点的距离为 ,此处需要调用数学函数sqrt(n)和pow(x,y)
时间: 2024-10-27 08:04:52 浏览: 27
要计算两点A(x1, y1)和B(x2, y2)之间的距离,你可以使用勾股定理,公式如下:
distance = sqrt((x2 - x1)^2 + (y2 - y1)^2)
在这个公式中,`sqrt()` 是平方根函数,用于计算平方的结果,`^` 表示幂运算。在C语言中,没有内置的 `sqrt()` 和 `pow()` 函数,但我们可以自己实现它们。以下是一个简单的C语言程序,包含了这两个函数以及计算两点距离的功能:
```c
#include <stdio.h>
#include <math.h>
// 自定义的平方根函数,使用牛顿法实现
double my_sqrt(double num) {
if (num == 0 || num == 1)
return num;
double guess = num / 2.0;
while (guess * guess > num) {
guess = (guess + num / guess) / 2.0;
}
return guess;
}
// 自定义的幂函数
double my_pow(double base, int exponent) {
double result = 1.0;
for (int i = 0; i < exponent; i++) {
result *= base;
}
return result;
}
int main() {
double x1, y1, x2, y2;
printf("Enter the coordinates of point A (x1, y1): ");
scanf("%lf %lf", &x1, &y1);
printf("Enter the coordinates of point B (x2, y2): ");
scanf("%lf %lf", &x2, &y2);
// 计算两点间的距离
double distance = my_sqrt(my_pow(x2 - x1, 2) + my_pow(y2 - y1, 2));
printf("The distance between points A and B is: %.2f\n", distance);
return 0;
}
```
这段代码首先定义了自定义的 `my_sqrt` 和 `my_pow` 函数,然后获取用户输入的点A和点B的坐标,最后计算并打印出两点之间的距离。
阅读全文
相关推荐
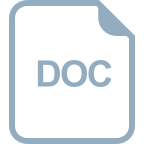
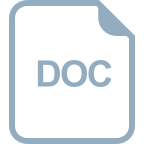
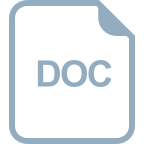

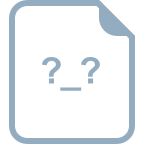
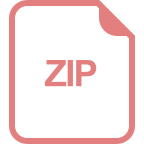
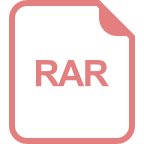
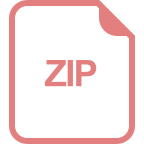
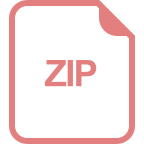
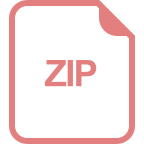
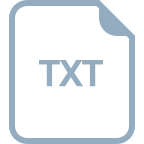
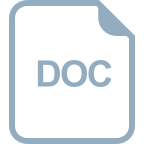
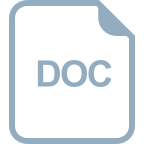
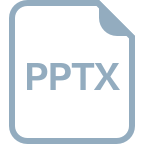
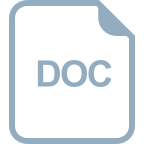
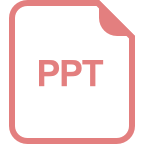
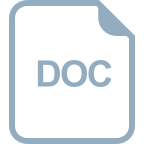