MultipartFile下载文件
时间: 2023-10-12 21:02:42 浏览: 70
在Java中,如果你想要使用MultipartFile来下载文件,可以按照以下步骤进行操作:
1. 首先,确保你已经导入了相关的依赖,包括Spring Web和Apache Commons IO。在Maven项目中,你可以在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.11.0</version>
</dependency>
```
2. 在你的控制器方法中,使用`@RequestParam`注解获取MultipartFile对象,该对象代表了要下载的文件。例如:
```java
@PostMapping("/download")
public ResponseEntity<Resource> downloadFile(@RequestParam("file") MultipartFile file) {
// 下载文件的逻辑
}
```
3. 在方法体中,你可以使用`Resource`接口来表示要下载的文件。你可以使用`ByteArrayResource`或`FileSystemResource`等实现类来创建一个Resource对象。例如:
```java
@PostMapping("/download")
public ResponseEntity<Resource> downloadFile(@RequestParam("file") MultipartFile file) {
// 创建一个Resource对象,表示要下载的文件
Resource resource = new ByteArrayResource(file.getBytes());
// 设置文件下载的响应头
HttpHeaders headers = new HttpHeaders();
headers.add(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=" + file.getOriginalFilename());
// 返回包含文件内容和响应头的ResponseEntity对象
return ResponseEntity.ok()
.headers(headers)
.contentLength(file.getSize())
.contentType(MediaType.APPLICATION_OCTET_STREAM)
.body(resource);
}
```
在上述代码中,我们使用`ByteArrayResource`来创建一个Resource对象,并将MultipartFile对象的内容作为字节数组传递给它。然后,我们设置了文件下载的响应头,包括`Content-Disposition`头,以指定文件名。最后,我们使用`ResponseEntity`来构建响应对象,并将Resource对象作为响应体返回。
这样,当你发起一个POST请求到`/download`接口,并传递一个名为`file`的文件参数时,服务器将会返回一个包含文件内容的响应,浏览器会自动下载该文件。
阅读全文
相关推荐
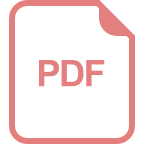















