import cv2 import numpy as np depth_image = cv2.imread('f.png', cv2.IMREAD_UNCHANGED) depth_image = depth_image / 1000.0 cv2.imshow('Depth Image', depth_image) cv2.waitKey(0) # 初始化灰度图像,注意这里创建的是单通道的8位灰度图像 Gray = np.zeros((depth_image.shape[0], depth_image.shape[1]), dtype=np.uint8) # 最大最小深度值 max = 255 # 注意:如果原深度图像只有8位,则应该将其设为255 min = 0 # 遍历每个像素,并进行深度值映射 for i in range(depth_image.shape[0]): data_gray = Gray[i] data_src = depth_image[i] for j in range(depth_image.shape[1]): if data_src[j] < max and data_src[j] > min: data_gray[j] = int((data_src[j] - min) / (max - min) * 255.0) else: data_gray[j] = 255 # 深度值不在范围内的置为白色 # 输出灰度图像,并保存 cv2.imwrite('/home/witney/test/0.jpg', Gray) cv2.imshow('gray', Gray) cv2.waitKey(0) #对图像进行二值化处理以便于轮廓检测 ret, thresh = cv2.threshold(Gray, 127, 255, cv2.THRESH_BINARY) cv2.imshow('thresh', thresh) cv2.waitKey(0) # 读取文本文件中的坐标位置信息 with open('f.txt', 'r') as f: positions = [] for line in f.readlines(): x1, y1, x2, y2 = map(float, line.strip().split(' ')) positions.append((x1, y1, x2, y2)) # 循环遍历每个坐标位置信息,绘制矩形框并截取图片内容 for i, pos in enumerate(positions): x1, y1, x2, y2 = pos # 根据坐标位置信息绘制矩形框 cv2.rectangle(thresh, (x1, y1), (x2, y2), (0, 255, 0), 2) # 利用数组切片功能截取图片中的内容 crop_img = thresh[y1:y2, x1:x2] # 保存截取的图片 cv2.imwrite(f'crop_image_{i}.jpg', crop_img)
时间: 2024-03-02 07:51:21 浏览: 17
这段代码中,第一行import cv2后面应该有一个空格,否则会出现语法错误。此外,如果您的f.png文件是16位深度图像,则需要使用cv2.IMREAD_UNCHANGED标志来读取,否则会被默认解释为8位深度图像。
另外,代码中的“max”变量应该命名为“max_depth”或类似的名称,以避免与Python内置函数“max()”发生冲突。
最后,你应该在程序的结尾处添加cv2.destroyAllWindows()语句,以确保所有的OpenCV窗口都已关闭。
修改后的代码如下:
```python
import cv2
import numpy as np
depth_image = cv2.imread('f.png', cv2.IMREAD_UNCHANGED) # 读取16位深度图像
depth_image = depth_image / 1000.0
cv2.imshow('Depth Image', depth_image)
cv2.waitKey(0)
# 初始化灰度图像,注意这里创建的是单通道的8位灰度图像
Gray = np.zeros((depth_image.shape[0], depth_image.shape[1]), dtype=np.uint8)
# 最大最小深度值
max_depth = 255 # 注意:如果原深度图像只有8位,则应该将其设为255
min_depth = 0
# 遍历每个像素,并进行深度值映射
for i in range(depth_image.shape[0]):
data_gray = Gray[i]
data_src = depth_image[i]
for j in range(depth_image.shape[1]):
if data_src[j] < max_depth and data_src[j] > min_depth:
data_gray[j] = int((data_src[j] - min_depth) / (max_depth - min_depth) * 255.0)
else:
data_gray[j] = 255 # 深度值不在范围内的置为白色
# 输出灰度图像,并保存
cv2.imwrite('/home/witney/test/0.jpg', Gray)
cv2.imshow('gray', Gray)
cv2.waitKey(0)
#对图像进行二值化处理以便于轮廓检测
ret, thresh = cv2.threshold(Gray, 127, 255, cv2.THRESH_BINARY)
cv2.imshow('thresh', thresh)
cv2.waitKey(0)
# 读取文本文件中的坐标位置信息
with open('f.txt', 'r') as f:
positions = []
for line in f.readlines():
x1, y1, x2, y2 = map(float, line.strip().split(' '))
positions.append((x1, y1, x2, y2))
# 循环遍历每个坐标位置信息,绘制矩形框并截取图片内容
for i, pos in enumerate(positions):
x1, y1, x2, y2 = pos
# 根据坐标位置信息绘制矩形框
cv2.rectangle(thresh, (int(x1), int(y1)), (int(x2), int(y2)), (0, 255, 0), 2)
# 利用数组切片功能截取图片中的内容
crop_img = thresh[int(y1):int(y2), int(x1):int(x2)]
# 保存截取的图片
cv2.imwrite(f'crop_image_{i}.jpg', crop_img)
cv2.destroyAllWindows()
```
相关推荐
















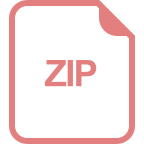
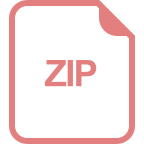