21点小游戏Python详细讲解
时间: 2023-06-15 18:04:34 浏览: 71
21点是一种常见的纸牌游戏,也叫做“Blackjack”。在这个游戏中,玩家需要比庄家更靠近21分,但不能超过21分。如果玩家手中的牌点数超过21分,则玩家输掉游戏。如果庄家手中的牌点数超过21分,则庄家输掉游戏。如果玩家手中的牌点数比庄家更靠近21分,则玩家赢得游戏。
下面我们来详细讲解一下如何使用 Python 编写一个简单的 21 点游戏。
1. 初始化游戏
首先,我们需要引入随机数模块和初始化一些变量。我们需要一副牌(52张),每张牌都有一个点数和花色。点数分别为1-10、J、Q、K,花色为红桃、方块、梅花、黑桃。我们使用一个列表来存储牌的点数和花色,再使用一个列表来存储已经发出的牌。
```python
import random
suits = ['Hearts', 'Diamonds', 'Clubs', 'Spades']
ranks = ['Ace', '2', '3', '4', '5', '6', '7', '8', '9', '10', 'Jack', 'Queen', 'King']
deck = []
for suit in suits:
for rank in ranks:
deck.append(f'{rank} of {suit}')
random.shuffle(deck)
player_hand = []
dealer_hand = []
player_score = 0
dealer_score = 0
```
2. 发牌
我们需要发两张牌给玩家和庄家。玩家可以看到自己的牌,但只能看到庄家的一张牌。
```python
for i in range(2):
player_card = deck.pop()
player_hand.append(player_card)
player_score += get_card_value(player_card)
dealer_card = deck.pop()
dealer_hand.append(dealer_card)
dealer_score += get_card_value(dealer_card)
print(f'Player has: {player_hand} ({player_score})')
print(f'Dealer has: {dealer_hand[0]}')
```
3. 判断是否胜利
如果玩家发牌后就直接达到了21点,则玩家赢了游戏。如果庄家发牌后就直接达到了21点,则庄家赢了游戏。
```python
if player_score == 21:
print('Player wins!')
return
if dealer_score == 21:
print('Dealer wins!')
return
```
4. 玩家操作
如果玩家没有直接达到21点,则需要询问玩家是否要继续要牌。如果玩家要牌,则再发一张牌,并计算总点数。如果玩家点数超过21点,则玩家输了游戏。
```python
while True:
action = input('Do you want to hit or stand? ')
if action.lower() == 'hit':
card = deck.pop()
player_hand.append(card)
player_score += get_card_value(card)
print(f'Player has: {player_hand} ({player_score})')
if player_score > 21:
print('Player busts! Dealer wins!')
return
else:
break
```
5. 庄家操作
如果玩家不再要牌后,则庄家需要继续要牌,直到庄家的点数超过17点或者爆牌。
```python
while dealer_score < 17:
card = deck.pop()
dealer_hand.append(card)
dealer_score += get_card_value(card)
print(f'Dealer has: {dealer_hand} ({dealer_score})')
if dealer_score > 21:
print('Dealer busts! Player wins!')
return
```
6. 判断胜负
如果玩家和庄家都没有爆牌,则比较点数大小,点数更接近21点的一方获胜。
```python
if player_score > dealer_score:
print('Player wins!')
elif player_score < dealer_score:
print('Dealer wins!')
else:
print('It\'s a tie!')
```
7. 完整代码
```python
import random
suits = ['Hearts', 'Diamonds', 'Clubs', 'Spades']
ranks = ['Ace', '2', '3', '4', '5', '6', '7', '8', '9', '10', 'Jack', 'Queen', 'King']
deck = []
for suit in suits:
for rank in ranks:
deck.append(f'{rank} of {suit}')
random.shuffle(deck)
player_hand = []
dealer_hand = []
player_score = 0
dealer_score = 0
def get_card_value(card):
if card.startswith('Ace'):
return 11
elif card.startswith('King') or card.startswith('Queen') or card.startswith('Jack'):
return 10
else:
return int(card.split()[0])
for i in range(2):
player_card = deck.pop()
player_hand.append(player_card)
player_score += get_card_value(player_card)
dealer_card = deck.pop()
dealer_hand.append(dealer_card)
dealer_score += get_card_value(dealer_card)
print(f'Player has: {player_hand} ({player_score})')
print(f'Dealer has: {dealer_hand[0]}')
if player_score == 21:
print('Player wins!')
elif dealer_score == 21:
print('Dealer wins!')
else:
while True:
action = input('Do you want to hit or stand? ')
if action.lower() == 'hit':
card = deck.pop()
player_hand.append(card)
player_score += get_card_value(card)
print(f'Player has: {player_hand} ({player_score})')
if player_score > 21:
print('Player busts! Dealer wins!')
break
else:
while dealer_score < 17:
card = deck.pop()
dealer_hand.append(card)
dealer_score += get_card_value(card)
print(f'Dealer has: {dealer_hand} ({dealer_score})')
if dealer_score > 21:
print('Dealer busts! Player wins!')
break
else:
if player_score > dealer_score:
print('Player wins!')
elif player_score < dealer_score:
print('Dealer wins!')
else:
print('It\'s a tie!')
break
```
相关推荐
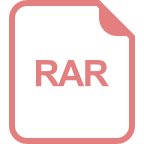














