#include <iostream> #include <string> using namespace std; int main() { int n; cin >> n; string s; getline(cin, s); // 读取换行符 for (int i = 0; i < n; i++) { getline(cin, s); int len = s.size(); int count = len * (len + 1) / 2; // 计算子串个数 cout << count << endl; } return 0; }
时间: 2024-04-26 18:22:15 浏览: 18
这段代码的功能是读入多个字符串,计算它们的子串个数并输出。具体来说,它的实现过程如下:
1. 首先读入一个整数 `n`,表示字符串的个数。
2. 然后使用 `getline` 函数读取每个字符串,并计算它的子串个数。
3. 由于一个长度为 `len` 的字符串有 `len*(len+1)/2` 个子串,所以我们可以直接计算出子串个数。
4. 最后输出结果。
需要注意的是,由于在读取 `n` 的时候使用了 `cin`,而 `cin` 会在读取一个整数后留下一个换行符,所以在读取每个字符串之前需要使用 `getline(cin, s)` 读取这个换行符。
如果你还有其他问题,请继续提问。
相关问题
#include<iostream> #include<string> using namespace std; int main(){ string str[5]; }
上述代码定义了一个大小为5的字符串数组`str`,每个元素都是一个`std::string`类型的字符串。
需要注意的是,在使用`std::string`类型的字符串时,我们无需担心字符串的长度问题,因为`std::string`会自动管理字符串的内存空间,可以动态地调整字符串的大小。因此,我们可以将任意长度的字符串赋值给数组中的元素,而不用担心数组元素的大小是否足够。
下面是一个示例,演示了如何使用`std::string`类型的字符串数组:
```c++
#include <iostream>
#include <string>
int main() {
// 定义一个字符串数组
std::string str[5];
// 从标准输入读取5个字符串,并存储到字符串数组中
for (int i = 0; i < 5; i++) {
std::cout << "请输入第" << i+1 << "个字符串:";
std::cin >> str[i];
}
// 遍历并输出字符串数组的每一个元素
for (int i = 0; i < 5; i++) {
std::cout << "第" << i+1 << "个字符串是:" << str[i] << std::endl;
}
return 0;
}
```
在上面的示例中,我们首先定义了一个大小为5的字符串数组`str`,然后使用for循环从标准输入读取5个字符串,并存储到字符串数组中。最后,我们再次使用for循环遍历字符串数组的每一个元素,并输出它们的值。
统一文件名只用#include<iostream> #include<string.h>
这两个头文件的作用不同,<iostream> 是用于输入输出流的库,而<string.h> 是用于字符串处理的库。如果你的程序中需要使用字符串相关函数,如strcpy、strlen等,就需要用到<string.h>头文件。如果要使用cout和cin等输入输出流对象则需要使用<iostream>头文件。如果你两个都需要用到,那么你就需要同时包含这两个头文件。例如:
```cpp
#include <iostream>
#include <string.h>
using namespace std;
int main() {
char str1[10] = "hello";
char str2[10] = "world";
strcat(str1, str2); // 使用<string.h>中的字符串拼接函数strcat
cout << str1 << endl; // 使用<iostream>中的输出流对象cout
return 0;
}
```
相关推荐
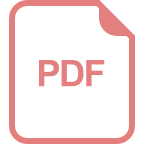
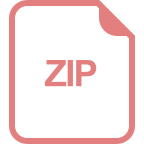
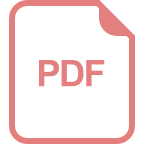














