python爬取微博评论
时间: 2023-10-05 14:08:54 浏览: 57
以下是使用 Python 爬取微博评论的步骤:
1. 登录微博开发平台,创建应用并获取 `APP_KEY` 和 `APP_SECRET`。
2. 安装 `weibo-sdk` 库,用于调用微博 API。
```
pip install weibo-sdk
```
3. 获取 OAuth2.0 授权,获取 `access_token`。
```python
import weibo
APP_KEY = 'your_app_key'
APP_SECRET = 'your_app_secret'
REDIRECT_URI = 'http://www.example.com/callback'
client = weibo.APIClient(app_key=APP_KEY, app_secret=APP_SECRET, redirect_uri=REDIRECT_URI)
auth_url = client.get_authorize_url()
print(auth_url)
code = input('请输入 code:')
r = client.request_access_token(code)
access_token = r.access_token
expires_in = r.expires_in
client.set_access_token(access_token, expires_in)
```
4. 调用 `comments/show` 接口获取评论列表。可以使用 `since_id` 和 `max_id` 参数控制翻页。
```python
import json
# 微博 ID
STATUS_ID = '1234567890'
# 获取前 200 条评论
page = 1
count = 200
since_id = None
max_id = None
comments = []
while True:
r = client.comments.show.get(id=STATUS_ID, count=count, page=page, since_id=since_id, max_id=max_id)
data = r.comments
if not data:
break
comments.extend(data)
max_id = data[-1].id
if len(comments) >= count:
break
print(json.dumps(comments, ensure_ascii=False, indent=4))
```
5. 对评论进行分析和处理。可以使用第三方库(如 `jieba`)进行分词和情感分析等操作。
```python
import jieba
import jieba.analyse
from snownlp import SnowNLP
# 分词
jieba.setLogLevel(20)
jieba.enable_paddle()
jieba.load_userdict('userdict.txt')
words = []
for comment in comments:
text = comment.text
seg_list = jieba.lcut(text, use_paddle=True)
words.extend(seg_list)
# 关键词提取
keywords = jieba.analyse.extract_tags(' '.join(words), topK=10, withWeight=True, allowPOS=('n', 'nr', 'ns', 'nt', 'nz', 'vn', 'v'))
# 情感分析
sentiments = []
for comment in comments:
text = comment.text
s = SnowNLP(text)
sentiments.append(s.sentiments)
# 输出结果
print('关键词:')
for keyword in keywords:
print(keyword[0], keyword[1])
print('情感分析:')
for sentiment in sentiments:
print(sentiment)
```
以上就是使用 Python 爬取微博评论的基本步骤。需要注意的是,微博 API 的使用需要遵守相关规定,不要进行违法和不良操作。
相关推荐











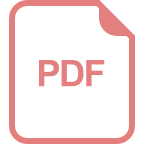
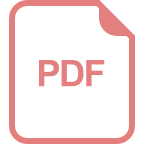