python编写ssm的代码
时间: 2023-07-23 08:17:51 浏览: 63
下面是一个使用Python编写状态空间模型(SSM)的例子,以ARMA(2,1)模型为例:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy import stats
# 构造ARMA(2,1)模型
phi = np.array([0.8, -0.7])
theta = np.array([0.5])
sigma = 1
T = 100
# 生成ARMA(2,1)模型数据
eps = stats.norm.rvs(loc=0, scale=sigma, size=T)
y = np.zeros(T)
y[0] = 0
y[1] = 0
for t in range(2, T):
y[t] = phi[0]*y[t-1] + phi[1]*y[t-2] + eps[t] + theta[0]*eps[t-1]
# 构建状态空间模型
from pykalman import KalmanFilter
kf = KalmanFilter(transition_matrices=np.array([[phi[0], phi[1]], [1, 0]]),
observation_matrices=np.array([[1, theta[0]]]),
transition_covariance=sigma**2 * np.eye(2),
observation_covariance=1,
initial_state_mean=np.zeros(2),
initial_state_covariance=np.eye(2),
n_dim_obs=1)
# 进行滤波和预测
state_means, state_covs = kf.filter(y)
filtered_means = state_means[:, 0]
filtered_stds = np.sqrt(state_covs[:, 0, 0])
predicted_means, predicted_covs = kf.filter_predict(y)
predicted_means = predicted_means[:, 0]
predicted_stds = np.sqrt(predicted_covs[:, 0, 0])
# 绘制结果
plt.plot(y, 'k.', label='Observations')
plt.plot(filtered_means, 'b-', label='Filtered')
plt.fill_between(np.arange(T), filtered_means - 2*filtered_stds, filtered_means + 2*filtered_stds,
color='b', alpha=0.2)
plt.plot(predicted_means, 'r-', label='Predicted')
plt.fill_between(np.arange(T), predicted_means - 2*predicted_stds, predicted_means + 2*predicted_stds,
color='r', alpha=0.2)
plt.legend(loc='lower right')
plt.show()
```
该例子使用了Python的pykalman库,该库提供了很方便的SSM建模和滤波/预测功能。在上述代码中,我们首先构造了一个ARMA(2,1)模型,并生成了100个样本。然后,我们使用pykalman库构建了一个状态空间模型,其中状态转移矩阵、观测矩阵、噪声方差等都需要手动设置。最后,我们使用滤波和预测函数来计算状态序列的后验均值和方差,并将结果绘制出来以进行可视化。
相关推荐
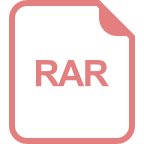
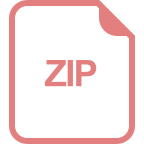
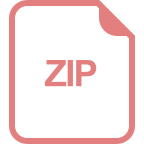














