实现RSA加密过程C++
时间: 2024-10-20 07:07:11 浏览: 27
RSA是一种非对称加密算法,它的基础是两个大素数的乘积的分解难题。以下是C++中实现RSA加密的基本步骤:
1. **密钥生成**:
- 选择两个大素数p和q。
- 计算n=p*q,作为公钥的模数。
- 求欧拉函数φ(n)=(p-1)*(q-1)。
- 选取一个小于φ(n)的整数e,满足gcd(e, φ(n))=1,这将用于公共密钥。
- 计算私钥d,使得(d*e)%φ(n)=1。
2. **加密** (公钥):
- 对明文m取模n,确保m < n。
- 使用模指数运算,计算c = m^e mod n,得到密文。
3. **解密** (私钥):
- 取密文c和公钥的模数n,使用私钥d计算m = c^d mod n。
4. **安全性关键**:
- 私钥通常不会公开,因为它允许数据的解密。只有持有私钥的人才能读取密文。
- 公钥可以广泛分发,任何人都能对消息进行加密,但只有拥有对应私钥的人才能解密。
下面是一个简单的C++代码片段展示了加密和解密的过程,注意实际应用中需要处理更复杂的错误边界和输入验证:
```cpp
#include <openssl/bio.h>
#include <openssl/pem.h>
#include <openssl/rsa.h>
// 加密部分
std::string encrypt(const std::string& plaintext, RSA* public_key) {
int len;
unsigned char ciphertext[1024];
BIO* bioOut = BIO_new(BIO_s_mem());
RSA_public_encrypt(plaintext.size(), reinterpret_cast<const unsigned char*>(plaintext.c_str()), ciphertext, public_key, RSA_PKCS1_OAEP_PADDING);
len = BIO_get_mem_data(bioOut, &ciphertext);
return std::string(reinterpret_cast<char*>(ciphertext), len);
}
// 解密部分
std::string decrypt(const std::string& ciphertext, RSA* private_key) {
int len;
unsigned char plaintext[1024];
BIO* bioIn = BIO_new_mem_buf(ciphertext.data(), static_cast<int>(ciphertext.length()));
RSA_private_decrypt(len, BIO_get_mem_ptr(bioIn), plaintext, private_key, RSA_PKCS1_OAEP_PADDING);
BIO_free_all(bioIn);
return std::string(reinterpret_cast<char*>(plaintext), len);
}
```
阅读全文
相关推荐
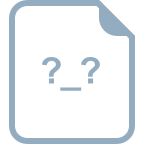
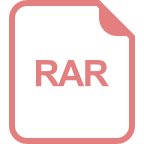
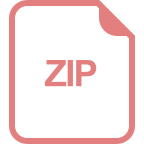
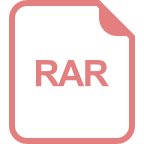
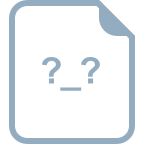
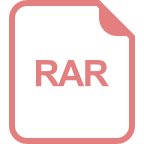
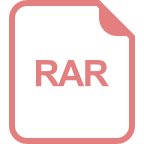
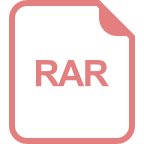
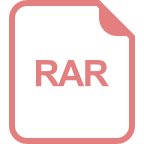
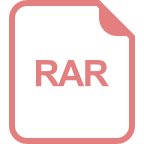
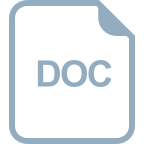





